Table of Contents
If you’re venturing into robotics and IoT development, combining the ESP8266 NodeMCU with a line tracking module is a powerful way to create intelligent and autonomous robots. This integration is ideal for building line-following robots, widely used in automation, education, and industrial applications.
In this guide, you’ll learn everything about setting up the ESP8266 NodeMCU with a line tracking sensor, including wiring, code, calibration, and enhancements to build a robust and intelligent robot.
The ESP8266 NodeMCU is a low-cost, Wi-Fi-enabled microcontroller that supports IoT applications and robotics. You can review its key specifications and setup guide here. On the other side, line tracking sensors such as the KY-033 or QTR-8RC detect changes in surface contrast (typically black vs. white) and allow a robot to follow a predefined path. If you’re interested in a practical project, this tutorial on using a line tracking sensor with ESP8266 is a great starting point.
What is the ESP8266 NodeMCU?
The ESP8266 NodeMCU is an open-source firmware and development board designed for Wi-Fi-based IoT projects. It is programmable via the Arduino IDE or other platforms like MicroPython and Lua.
Key Features:
- Integrated Wi-Fi connectivity
- GPIO pins for external components
- Compact size with USB interface
- Compatible with 3.3V logic
Applications:
- Smart home devices
- Sensor data collection
- Wireless robots
- Home automation systems
Understanding Line Tracking Modules
Line tracking modules use infrared sensors to detect changes in reflectivity between black and white surfaces. When a robot is placed on a surface with a dark line, the sensor can guide the robot to follow the path.
Common Modules:
- KY-033 Infrared Obstacle Avoidance
- QTR-8RC Reflectance Sensor Array
How They Work:
- IR LED emits light
- Photodiode measures reflection
- Low reflection = black line
- High reflection = white surface
Applications:
- Line-following robots
- Automated guided vehicles (AGVs)
- Maze-solving bots
Wiring ESP8266 NodeMCU with Line Tracking Module
Connecting the ESP8266 NodeMCU to a line tracking module is straightforward. You’ll typically use the digital output of the sensor to read line detection signals.
Components Needed:
- 1x ESP8266 NodeMCU
- 1x Line tracking sensor (e.g., KY-033)
- Jumper wires
- Breadboard or chassis
Pin Connections:
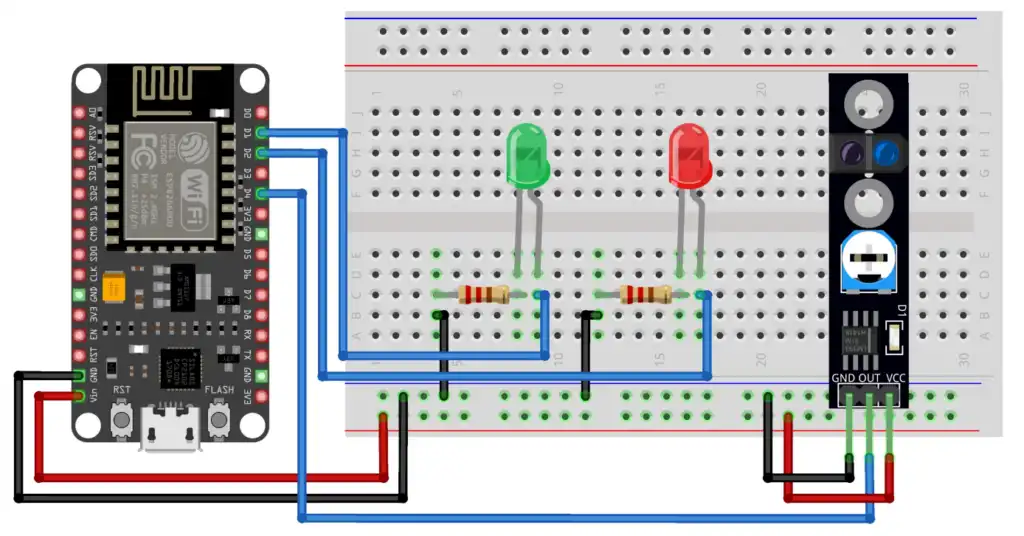
- VCC → 3.3V on ESP8266
- GND → GND on ESP8266
- OUT → GPIO (e.g., D4)
Sample Code (Arduino IDE):
cppCopyEditint sensorPin = D4;
void setup() {
pinMode(sensorPin, INPUT);
Serial.begin(9600);
}
void loop() {
int value = digitalRead(sensorPin);
Serial.println(value);
delay(100);
}
Building a Line-Following Robot with ESP8266
Creating a robot that follows a line involves integrating the sensor with motor drivers and power management.
Step-by-Step Guide:
- Attach motors to the chassis
- Connect ESP8266 to motor driver (e.g., L298N)
- Mount the line tracking module at the front
- Upload the line-following code
- Test on a black line over a white surface
Control Logic:
- Sensor detects black line: Move forward
- Left sensor off line: Turn right
- Right sensor off line: Turn left
For more advanced control, consider this Wi-Fi robot car tutorial with ESP8266.
Enhancing with Advanced Features
You can take your robot beyond basic functionality using smart algorithms and additional sensors.
Improvements:
- PID Control: Implement proportional-integral-derivative control for smoother navigation (PID line follower robot guide)
- Wi-Fi Control: Use a smartphone app or web interface to remotely control the robot
- Sensor Fusion: Add ultrasonic or IR sensors for obstacle avoidance
- Speed Modulation: Adjust motor speed based on curve detection
Troubleshooting Common Issues
Even with a well-planned setup, you might run into a few common problems:
Sensor Not Detecting Line:
- Ensure correct VCC (3.3V) connection
- Verify the sensor is aligned close to the surface
Wi-Fi Connection Fails:
- Check SSID and password
- Reboot and reflash firmware
Robot Drifts Off Line:
- Adjust sensor height and angle
- Tune the threshold value in code
Power Issues:
- Use a stable 5V power source for motors
- Ensure ESP8266 gets a steady 3.3V
Frequently Asked Questions (FAQs)
What is the difference between KY-033 and QTR-8RC?
KY-033 is a single-sensor module ideal for simple projects, while QTR-8RC offers multiple sensors for more accurate tracking.
Can ESP8266 work with Arduino components?
Yes, it’s compatible with most Arduino-compatible modules and can be programmed using the Arduino IDE.
How do I calibrate the line tracking sensor?
Use the onboard potentiometer (if available) to fine-tune the sensitivity depending on your surface.
Can I control my robot using a smartphone?
Yes, the ESP8266’s Wi-Fi functionality allows remote control via a custom app or web interface.
Is the ESP8266 suitable for complex robots?
It’s great for basic to moderate robotics but may have limitations in processing power for advanced real-time control.
Conclusion
Combining the ESP8266 NodeMCU with a line tracking module offers a powerful yet beginner-friendly way to dive into robotics, IoT, and embedded systems. This setup enables the development of a line-following robot that can detect and react to its environment using infrared sensors, making it an ideal project for students, hobbyists, and tech enthusiasts.
The ESP8266 brings in the added advantage of Wi-Fi connectivity, allowing for remote monitoring, control, and even data logging through IoT platforms. Whether you’re just getting started or looking to level up your robotics knowledge, integrating the ESP8266 with sensors, motor drivers, and control algorithms provides a hands-on, scalable learning experience.
As you explore further, consider enhancing your project with PID control, obstacle detection, or even mobile app integration. These additions can make your robot more accurate, autonomous, and functional in complex scenarios.
This project not only teaches core principles like circuit design, sensor integration, and embedded programming but also opens the door to more advanced innovations in automation. Keep experimenting with different modules and techniques—there’s virtually no limit to what you can build with this versatile microcontroller and sensor combo.
Projects ESP8266 nodemcu :
1- ESP8266 NodeMCU: A Comprehensive Guide to IoT Development
2- Control LED with ESP8266 NodeMCU: A Beginner’s Guide
3- Integrating a Joystick with ESP8266 NodeMCU: A Comprehensive Guide
4- esp8266 nodemcu with Flame Sensor
5- ESP8266 NodeMCU with Heartbeat Sensor
6- ESP8266 NodeMCU with KY-027 Magic Cup Light
7- ESP8266 NodeMCU with Hall Magnetic Sensor
8- ESP8266 NodeMCU with relay module 5v
9- ESP8266 NodeMCU with Linear Hall Sensor