Table of Contents
Introduction
Measuring wind speed is essential in fields like meteorology, agriculture, aviation, and renewable energy. With the rise of the Internet of Things (IoT), it’s now easier than ever to build a smart, connected weather station at home or for research purposes. One of the most affordable and powerful tools for this task is the ESP8266 NodeMCU with an Anemometer: Measure Wind Speed.
In this guide, we’ll show how to connect an anemometer to the ESP8266 NodeMCU to accurately measure wind speed, log data, and even send it to the cloud. This project combines basic electronics, coding, and IoT integration for an efficient DIY solution. A great step-by-step guide to working with the ESP8266 and wind sensors can be found in this ESP8266 anemometer tutorial by Random Nerd Tutorials.
For those looking to build their own anemometer from scratch, this Instructables DIY anemometer project offers an excellent foundation.
Understanding the Anemometer
What Is an Anemometer?
An anemometer is a device used to measure wind speed. There are different types:
- Cup anemometer (mechanical, most common)
- Vane anemometer (measures direction and speed)
- Hot-wire anemometer (uses heat loss to detect airflow)
- Ultrasonic anemometer (uses sound waves to determine speed)
How Does It Work?
The cup anemometer rotates as wind moves past it. Each rotation represents a specific amount of wind movement. The faster the wind, the more rotations occur, which can be translated into a voltage signal or pulse.
Technical Specifications
- Voltage output: typically 0V to 5V
- Signal: Analog or digital (depending on the model)
- Response time: milliseconds
- Wind speed range: up to 30 m/s for many consumer models
Overview of ESP8266 NodeMCU
Key Features
The ESP8266 NodeMCU is a low-cost Wi-Fi microcontroller module, perfect for IoT applications. It includes:
- Built-in Wi-Fi connectivity
- GPIO pins for sensor integration
- ADC (Analog-to-Digital Converter) for analog sensors
- USB for programming via Arduino IDE
Why Use It for Wind Speed Measurement?
- Extremely affordable
- Reliable for real-time data capture
- Supported by a large open-source community
- Easy integration with cloud platforms
Hardware Requirements
Components Needed
- ESP8266 NodeMCU board
- Anemometer sensor (analog output preferred)
- Breadboard and jumper wires
- Resistors for voltage divider
- 12V external power supply (for some anemometers)
Optional Add-ons
- OLED display for visual feedback
- Waterproof enclosure for outdoor use
- SD card module for local data logging
Circuit Design and Wiring
Power Supply
Most anemometers require 5V to 12V. If you’re using a 12V model, don’t power it directly from the ESP8266. Use an external 12V power source.
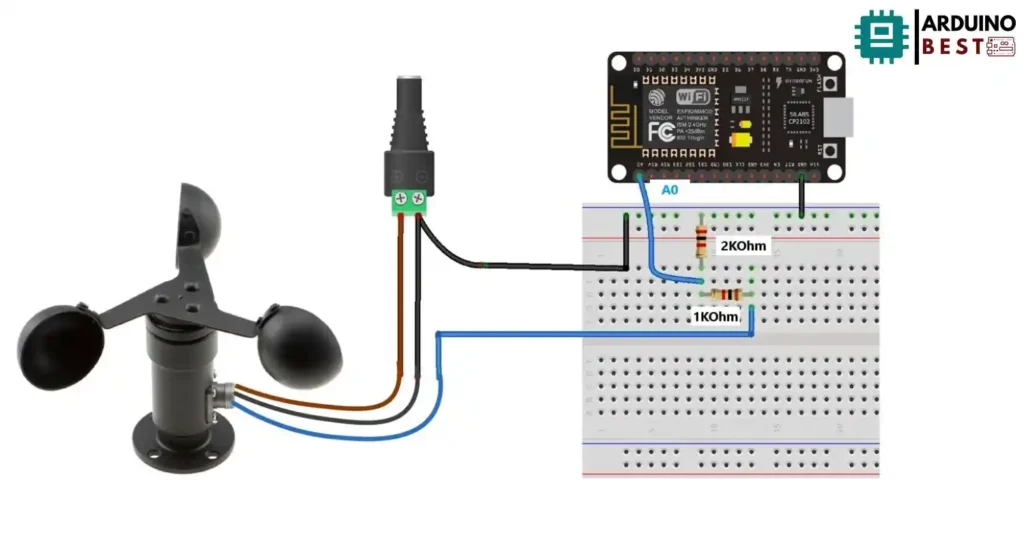
Voltage Divider
Because the ESP8266 ADC only handles up to 3.3V, a voltage divider is necessary to scale down the signal:
- Use two resistors (e.g., 10kΩ and 20kΩ) to divide voltage
- Connect analog output from the anemometer through the divider before feeding into the A0 pin
Wiring Schematic
- Anemometer power to 12V source
- Anemometer ground to common ground
- Anemometer signal to voltage divider, then to A0 pin
- ESP8266 powered via USB or regulated 3.3V
Programming the ESP8266
Arduino IDE Setup
- Install the ESP8266 board manager in the Arduino IDE
- Select “NodeMCU 1.0 (ESP-12E Module)”
- Install required libraries:
ESP8266WiFi
,Adafruit_GFX
,Adafruit_SSD1306
(for OLED)
Reading and Converting Data
- Use
analogRead(A0)
to read voltage - Map voltage range (e.g., 0.4V to 2.0V) to wind speed using calibration values
Example conversion:
// Constants (Change the following variables if needed)
const int anemometerPin = A0; // GPIO pin connected to anemometer (analog pin)
const float minVoltage = 0.033; // Voltage corresponding to 0 m/s
const float maxVoltage = 3.3; // Voltage corresponding to 32.4 m/s (max speed) (when using voltage divider)
const float maxWindSpeed = 32.4; // Maximum wind speed in m/s
// Conversion factors
const float mps_to_kmh = 3.6; // 1 m/s = 3.6 km/h
const float mps_to_mph = 2.23694; // 1 m/s = 2.23694 mph
void setup() {
Serial.begin(115200);
}
void loop() {
// Read analog value from anemometer (ADC value between 0-1023 on ESP8266 for 0-3.3V)
int adcValue = analogRead(anemometerPin);
// Convert ADC value to voltage (ESP8266 ADC range is 0-3.3V)
float voltage = (adcValue / 1023.00) * 3.3;
// Ensure the voltage is within the anemometer operating range
if (voltage < minVoltage) {
voltage = minVoltage;
} else if (voltage > maxVoltage) {
voltage = maxVoltage;
}
// Map the voltage to wind speed
float windSpeed_mps = ((voltage - minVoltage) / (maxVoltage - minVoltage)) * maxWindSpeed;
// Convert wind speed to km/h and mph
float windSpeed_kmh = windSpeed_mps * mps_to_kmh;
float windSpeed_mph = windSpeed_mps * mps_to_mph;
// Print wind speed
Serial.print("Wind Speed: ");
Serial.print(windSpeed_mps);
Serial.print(" m/s, ");
Serial.print(windSpeed_kmh);
Serial.print(" km/h, ");
Serial.print(windSpeed_mph);
Serial.println(" mph");
delay(1000);
}
Displaying Data
- Use
Serial.print()
for debugging - Optional: Output to OLED display or log to file
Calibration and Testing
Why Calibrate?
Different anemometers produce slightly different voltage responses, so calibration ensures accurate wind speed readings.
How to Calibrate
- Compare readings with a known accurate device or manufacturer chart
- Use a hairdryer or fan with a known wind speed setting to generate airflow
Adjusting the Formula
Once you gather enough data:
- Plot voltage vs. known speed
- Fit a linear or polynomial equation
- Update code to reflect this relationship
Data Logging and IoT Integration
Local Storage
- Use an SD card module to save data with timestamps
- Format data as CSV for easy import to spreadsheets
Cloud Services
- Send data to platforms like ThingSpeak or Blynk
- Use HTTP or MQTT protocols
Steps for ThingSpeak:
- Sign up for a free account
- Create a new channel with fields for wind speed
- Use API keys in your code to send updates
Real-Time Dashboards
- Display wind data on a web dashboard
- Use Node-RED, Grafana, or Google Sheets for visualization
Applications and Use Cases
- Home weather stations: Monitor wind speed in real-time
- Agricultural monitoring: Automate irrigation based on wind conditions
- Renewable energy assessments: Test wind turbine feasibility
- Sailing and sports: Provide accurate environmental data
Troubleshooting and Tips
- Ensure stable voltage supply to avoid noisy signals
- Avoid long cables between sensor and ESP8266 to minimize voltage drop
- Shield your setup from rain and direct sunlight
- Check your wiring connections if you get flat-line or noisy signals
FAQs
How do I calibrate my anemometer with ESP8266?
Use a reference wind source or another calibrated anemometer and match voltage outputs with known speeds.
Can I use a digital anemometer with the ESP8266?
Yes, if the sensor provides digital pulses, you can measure the frequency to determine wind speed.
What is the maximum wind speed this setup can measure?
It depends on the anemometer. Most consumer-grade models measure up to 30 m/s.
How do I protect my device from weather?
Use a waterproof enclosure, and seal openings with rubber grommets.
Can I use multiple sensors with one ESP8266?
Yes, but be aware of the limited ADC pin. Use I2C or multiplexers for additional analog sensors.
Conclusion
Creating a wind speed monitoring system using an ESP8266 NodeMCU and an anemometer is not only a cost-effective solution but also a powerful introduction to modern IoT applications. This project allows you to capture real-time wind speed data, display it locally, and transmit it to cloud platforms for remote monitoring and long-term analysis.
By understanding how to wire sensors correctly, process analog data, and calibrate sensor output, you build a foundation in both electronics and programming. Integrating with platforms like ThingSpeak or using an OLED display enhances your system’s capabilities and usability.
Whether you’re building a DIY weather station, supporting agricultural decision-making, or conducting environmental research, this system offers accuracy, flexibility, and expandability. With endless opportunities for customization, including automation or multi-sensor integration, this project serves as a launchpad into the broader world of embedded systems and connected devices.
Projects ESP8266 nodemcu :
1- ESP8266 NodeMCU: A Comprehensive Guide to IoT Development
2- Control LED with ESP8266 NodeMCU: A Beginner’s Guide
3- Integrating a Joystick with ESP8266 NodeMCU: A Comprehensive Guide
4- esp8266 nodemcu with Flame Sensor
5- ESP8266 NodeMCU with Heartbeat Sensor
6- ESP8266 NodeMCU with KY-027 Magic Cup Light
7- ESP8266 NodeMCU with Hall Magnetic Sensor
8- ESP8266 NodeMCU with relay module 5v
9- ESP8266 NodeMCU with Linear Hall Sensor