Table of Contents
Introduction
Accurate timekeeping is essential in various electronic projects, from data logging to scheduling tasks. The Arduino DS3231 Real Time Clock (RTC) module is one of the most reliable and precise solutions for maintaining accurate time even when power is lost. Unlike software-based clocks, RTC modules run independently and provide long-term accuracy with minimal drift.
In this article, we’ll explore how to interface the DS3231 RTC with Arduino, set the time, configure alarms, and use its advanced features. If you’re new to RTCs, you might want to check out this guide on I²C communication to understand how the module communicates with Arduino.
Understanding Real-Time Clocks (RTCs)
- Real-Time Clocks (RTCs) are hardware-based timekeeping modules that keep track of time even when the microcontroller is turned off.
- They use an oscillator and a battery backup to maintain time precision.
- Unlike software clocks, hardware RTCs are immune to resets and power failures.
Overview of the DS3231 RTC Module
The DS3231 is a high-accuracy RTC module with a built-in temperature-compensated crystal oscillator (TCXO) and an I²C interface.
Key Features:
- High Accuracy: Maintains precise time with an integrated crystal oscillator.
- Battery Backup: Ensures continued timekeeping during power loss.
- I²C Interface: Enables easy communication with Arduino and other microcontrollers.
- Alarm Functions: Supports two programmable alarms.
- Built-in Temperature Sensor: Measures the ambient temperature with a precision of ±3°C.
For a detailed comparison of RTC modules, check out this comparison of DS3231 vs DS1307.
Interfacing the DS3231 with Arduino
Required Components:
- Arduino board (Uno, Mega, Nano, etc.)
- DS3231 RTC module
- Jumper wires
Wiring Diagram:
DS3231 Pin | Arduino Pin |
---|---|
VCC | 5V |
GND | GND |
SDA | A4 |
SCL | A5 |
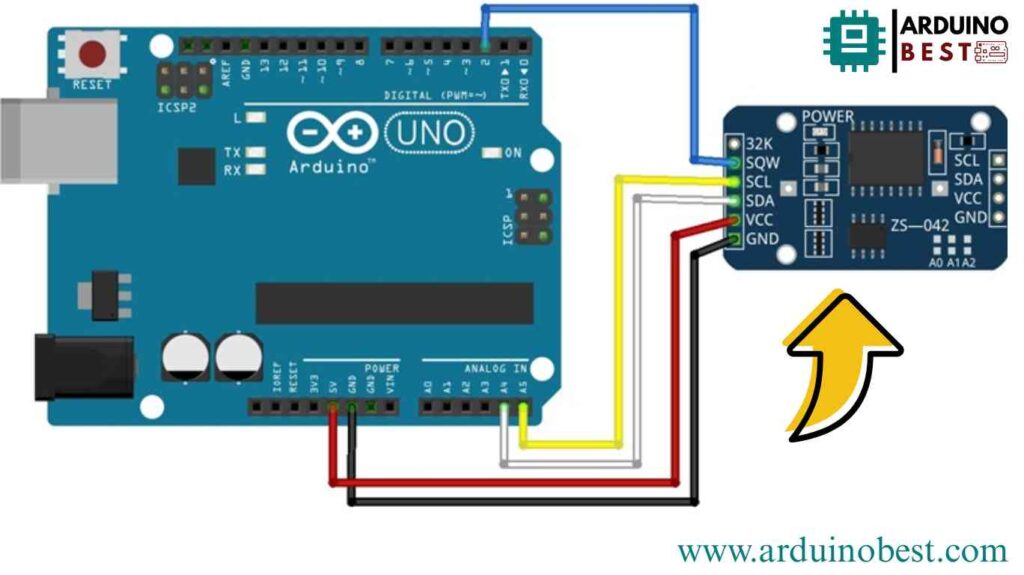
Setting Up the Arduino Development Environment
- Install the Arduino IDE from the official Arduino website.
- Install the Wire.h and DS3231.h libraries:
#include <Wire.h> #include <DS3231.h> DS3231 rtc;
Programming the DS3231 for Timekeeping
Setting the Time
rtc.setDOW(FRIDAY);
rtc.setTime(10, 30, 45); // Set time to 10:30:45
rtc.setDate(22, 3, 2025); // Set date to March 22, 2025
Reading the Time
Serial.println(rtc.getTimeStr());
Implementing Alarms with DS3231
- The DS3231 has two programmable alarms:
- Alarm 1 triggers based on hours, minutes, and seconds.
- Alarm 2 triggers based on hours and minutes only.
Setting an Alarm
rtc.setAlarm(ALM1_MATCH_SECONDS, 30, 10, 0, 0);
Clearing an Alarm
rtc.clearAlarm(1);
Advanced Features and Applications
- Temperature Monitoring:
Serial.println(rtc.getTemp());
- Square Wave Output for Timers:
rtc.enableSquareWave(1);
Troubleshooting Common Issues
- RTC not keeping time? Check the battery connection.
- Communication failure? Ensure SDA and SCL are correctly connected.
- Alarms not triggering? Verify the correct alarm mode is set.
code arduino
// Based on the RTCLib Library examples: https://github.com/adafruit/RTClib/blob/master/examples
// Date and time functions using a DS1307 RTC connected via I2C and Wire lib
#include "RTClib.h"
RTC_DS3231 rtc;
char daysOfTheWeek[7][12] = {"Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday"};
void setup () {
Serial.begin(9600);
if (! rtc.begin()) {
Serial.println("Couldn't find RTC");
Serial.flush();
while (1) delay(10);
}
if (! rtc.lostPower()) {
Serial.println("RTC lost power, let's set the time!");
// When time needs to be set on a new device, or after a power loss, the
// following line sets the RTC to the date & time this sketch was compiled
rtc.adjust(DateTime(F(__DATE__), F(__TIME__)));
// This line sets the RTC with an explicit date & time, for example to set
// January 21, 2014 at 3am you would call:
//rtc.adjust(DateTime(2014, 1, 21, 3, 0, 0));
}
// When time needs to be re-set on a previously configured device, the
// following line sets the RTC to the date & time this sketch was compiled
rtc.adjust(DateTime(F(__DATE__), F(__TIME__)));
// This line sets the RTC with an explicit date & time, for example to set
// January 21, 2014 at 3am you would call:
//rtc.adjust(DateTime(2014, 1, 21, 3, 0, 0));
}
void loop () {
// Get the current time from the RTC
DateTime now = rtc.now();
// Getting each time field in individual variables
// And adding a leading zero when needed;
String yearStr = String(now.year(), DEC);
String monthStr = (now.month() < 10 ? "0" : "") + String(now.month(), DEC);
String dayStr = (now.day() < 10 ? "0" : "") + String(now.day(), DEC);
String hourStr = (now.hour() < 10 ? "0" : "") + String(now.hour(), DEC);
String minuteStr = (now.minute() < 10 ? "0" : "") + String(now.minute(), DEC);
String secondStr = (now.second() < 10 ? "0" : "") + String(now.second(), DEC);
String dayOfWeek = daysOfTheWeek[now.dayOfTheWeek()];
// Complete time string
String formattedTime = dayOfWeek + ", " + yearStr + "-" + monthStr + "-" + dayStr + " " + hourStr + ":" + minuteStr + ":" + secondStr;
// Print the complete formatted time
Serial.println(formattedTime);
// Getting temperature
Serial.print(rtc.getTemperature());
Serial.println("ºC");
Serial.println();
delay(3000);
}
FAQs
How long does the DS3231 keep time without power?
With a coin cell battery, the RTC module can keep time for years without external power.
Can the DS3231 work with 3.3V microcontrollers?
Yes, the DS3231 operates within a 2.3V-5.5V range, making it compatible with 3.3V systems.
How accurate is the DS3231?
The DS3231 has an accuracy of ±2 minutes per year due to its temperature compensation.
Conclusion
The Arduino DS3231 Real Time Clock (RTC) module is an essential component for precise and reliable timekeeping. By integrating alarms and advanced features, it can be used in various applications like timers, scheduling, and logging. Experiment with the DS3231 in your projects and unlock its full potential!
Here we suggest other Arduino Projects:
1- Complete Guide for DHT11/DHT22 Humidity and Temperature Sensor With Arduino
2- DHT11 – Temperature and Humidity Sensor
3- DHT22 – Temperature and Humidity Sensor (more accurate than DHT11)
4- BMP180 – Barometric Pressure and Altitude Sensor
5- BMP280 – Barometric Pressure & Temperature Sensor
6- BME280 – Temperature, Humidity, and Pressure Sensor
7- Arduino Flex Sensor Controlled Robot Hand
8- Arduino ECG Heart Rate Monitor AD8232 Demo
9- Arduino NRF24L01 Wireless Joystick Robot Car
10- Arduino Force Sensor Anti-Theft Alarm System
11- Arduino NRF24L01 Transceiver Controlled Relay Light
12- Arduino Rotary Encoder Controlled LEDs: A Complete Guide