Table of Contents
Creating a digital spirit level using Arduino and the MPU6050 sensor is an exciting project that combines electronics, programming, and practical applications. The MPU6050 sensor is a combination of an accelerometer and a gyroscope, which makes it ideal for measuring tilt and orientation in 3D space. This project provides a DIY solution to a traditional tool, offering a more accurate and flexible way of measuring levels, whether you’re working in construction or calibration tasks.
In this guide, we’ll show you how to build a digital spirit level using the Arduino MPU6050 sensor, along with an OLED display or LCD to visualize the data. This is a fantastic project for beginners who want to learn more about sensors and Arduino, as well as those who want to make a practical, customizable measuring tool. If you’re unfamiliar with the MPU6050 sensor, you can start by checking out this in-depth guide on using the MPU6050 with Arduino to get a better understanding of its capabilities and how it works.
With a few simple components and some coding, you’ll be able to create a reliable level measurement system that you can use in various DIY projects. Whether you’re leveling furniture, calibrating equipment, or performing construction tasks, this digital spirit level can provide precise measurements.
What is the MPU6050?
The MPU6050 is a sensor that combines an accelerometer and a gyroscope on a single chip. The accelerometer measures static acceleration, including gravity, and the gyroscope measures the rate of rotation. Together, they provide a complete picture of the orientation of an object in three-dimensional space.
The MPU6050 sensor is commonly used in robotics, drones, and other motion-detecting applications. For this project, the sensor allows us to measure tilt angles along the X, Y, and Z axes, which is essential for creating a digital spirit level. Using the Arduino to process the data, we can display the tilt angle on an OLED or LCD screen.
If you’re looking for additional information on how the MPU6050 works and how it can be implemented in Arduino projects, check out this guide to Arduino IDE and libraries.
Components Required for the Project
Before diving into the assembly and coding, it’s essential to gather the components required for this project. Here’s a list of the key parts:
- Arduino board (Uno, Nano, etc.)
- MPU6050 sensor (Accelerometer and Gyroscope)
- OLED Display or LCD
- Breadboard and Jumper wires
- Resistors (if needed)
- Power supply (USB or external power)
- Optional: Buzzer, LEDs, and a 3D printed enclosure (for a neat finish)
Additionally, you will need the Arduino IDE installed on your computer, along with the necessary libraries such as Wire.h
, MPU6050.h
, and LiquidCrystal_I2C.h
for proper communication with the sensor and display.
Circuit Design and Assembly
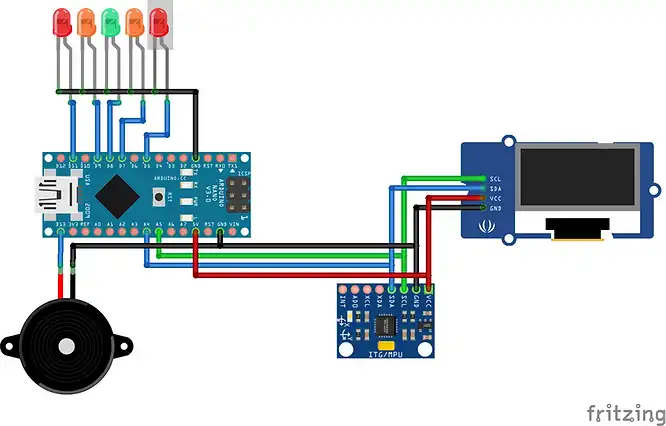
Now that you have your components, let’s focus on the circuit design. The MPU6050 communicates with the Arduino via the I2C interface, which uses only two pins: SCL (clock) and SDA (data). Here’s how to wire everything together:
- MPU6050 to Arduino:
- VCC to 5V
- GND to GND
- SCL to A5 (on Uno)
- SDA to A4 (on Uno)
- OLED/LCD to Arduino:
- VCC to 5V
- GND to GND
- SCL to A5
- SDA to A4
The power supply will come through the Arduino’s USB connection or an external source, depending on your setup.
Understanding the MPU6050 Data
To create an accurate digital spirit level, we need to read the data from the MPU6050 and convert it into angles. The accelerometer data provides the tilt in the X, Y, and Z axes, and by using simple trigonometry, we can calculate the tilt angles.
Once you start receiving data from the MPU6050, you’ll notice that it outputs raw sensor data. This data needs to be processed to give meaningful tilt angles. You can use the following formulas to calculate the angles:
- Pitch (X-axis tilt) =
atan(accelY / sqrt(accelX^2 + accelZ^2))
- Roll (Y-axis tilt) =
atan(-accelX / sqrt(accelY^2 + accelZ^2))
These angles can then be displayed on the OLED or LCD screen, showing the user the precise level measurement.
Arduino Code Implementation
Now let’s talk about the Arduino code. The first step is to include the necessary libraries and set up the MPU6050 sensor and the display. Below is an example of how to initialize the MPU6050 sensor:
#include <Wire.h>
#include <Adafruit_SSD1306.h>
//OLED====================================================================
#define SCREEN_WIDTH 128
#define SCREEN_HEIGHT 64
#define OLED_RESET 4
Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, OLED_RESET);
//Level LEDs==============================================================
int levelLED_neg2 = 5;
int levelLED_neg1 = 7;
int levelLED_level = 8;
int levelLED_pos1 = 9;
int levelLED_pos2 = 11;
int buzzer = 13;
//Variables for Gyroscope=================================================
int gyro_x, gyro_y, gyro_z;
long gyro_x_cal, gyro_y_cal, gyro_z_cal;
boolean set_gyro_angles;
long acc_x, acc_y, acc_z, acc_total_vector;
float angle_roll_acc, angle_pitch_acc;
float angle_pitch, angle_roll;
int angle_pitch_buffer, angle_roll_buffer;
float angle_pitch_output, angle_roll_output;
// Setup timers and temp variables
long loop_timer;
int temp;
// Display counter
int displaycount = 0;
//========================================================================
void setup()
{
Wire.begin();
Serial.begin(9600);
if (!display.begin(SSD1306_SWITCHCAPVCC, 0x3C))
{
Serial.println(F("SSD1306 allocation failed"));
for (;;); // Don't proceed, loop forever
}
display.clearDisplay();
analogReference(INTERNAL);
pinMode(levelLED_neg2, OUTPUT);
pinMode(levelLED_neg1, OUTPUT);
pinMode(levelLED_level, OUTPUT);
pinMode(levelLED_pos1, OUTPUT);
pinMode(levelLED_pos2, OUTPUT);
pinMode(buzzer, OUTPUT);
//Setup the registers of the MPU-6050
setup_mpu_6050_registers();
//Read the raw acc and gyro data from the MPU-6050 1000 times
for (int cal_int = 0; cal_int < 1000 ; cal_int ++)
{
read_mpu_6050_data();
//Add the gyro x offset to the gyro_x_cal variable
gyro_x_cal += gyro_x;
//Add the gyro y offset to the gyro_y_cal variable
gyro_y_cal += gyro_y;
//Add the gyro z offset to the gyro_z_cal variable
gyro_z_cal += gyro_z;
//Delay 3us to have 250Hz for-loop
delay(3);
}
// Divide all results by 1000 to get average offset
gyro_x_cal /= 1000; gyro_y_cal /= 1000; gyro_z_cal /= 1000;
Serial.begin(115200);
loop_timer = micros();
}
//========================================================================
void loop()
{
// Get data from MPU-6050
read_mpu_6050_data();
//Subtract the offset values from the raw gyro values
gyro_x -= gyro_x_cal;
gyro_y -= gyro_y_cal;
gyro_z -= gyro_z_cal;
//Gyro angle calculations . Note 0.0000611 = 1 / (250Hz x 65.5)
//Calculate the traveled pitch angle and add this to the angle_pitch variable
angle_pitch += gyro_x * 0.0000611;
//Calculate the traveled roll angle and add this to the angle_roll variable
//0.000001066 = 0.0000611 * (3.142(PI) / 180degr) The Arduino sin function is in radians
angle_roll += gyro_y * 0.0000611;
//If the IMU has yawed transfer the roll angle to the pitch angle
angle_pitch += angle_roll * sin(gyro_z * 0.000001066);
//If the IMU has yawed transfer the pitch angle to the roll angle
angle_roll -= angle_pitch * sin(gyro_z * 0.000001066);
//Accelerometer angle calculations
//Calculate the total accelerometer vector
acc_total_vector = sqrt((acc_x*acc_x)+(acc_y*acc_y)+(acc_z*acc_z));
//57.296 = 1 / (3.142 / 180) The Arduino asin function is in radians
//Calculate the pitch angle
angle_pitch_acc = asin((float)acc_y/acc_total_vector)* 57.296;
//Calculate the roll angle
angle_roll_acc = asin((float)acc_x/acc_total_vector)* -57.296;
//Accelerometer calibration value for pitch
angle_pitch_acc -= 0.0;
//Accelerometer calibration value for roll
angle_roll_acc -= 0.0;
if(set_gyro_angles)
{
//If the IMU has been running
//Correct the drift of the gyro pitch angle with the accelerometer pitch angle
angle_pitch = angle_pitch * 0.9996 + angle_pitch_acc * 0.0004;
//Correct the drift of the gyro roll angle with the accelerometer roll angle
angle_roll = angle_roll * 0.9996 + angle_roll_acc * 0.0004;
}
else
{
//IMU has just started
//Set the gyro pitch angle equal to the accelerometer pitch angle
angle_pitch = angle_pitch_acc;
//Set the gyro roll angle equal to the accelerometer roll angle
angle_roll = angle_roll_acc;
//Set the IMU started flag
set_gyro_angles = true;
}
//To dampen the pitch and roll angles a complementary filter is used
//Take 90% of the output pitch value and add 10% of the raw pitch value
angle_pitch_output = angle_pitch_output * 0.9 + angle_pitch * 0.1;
//Take 90% of the output roll value and add 10% of the raw roll value
angle_roll_output = angle_roll_output * 0.9 + angle_roll * 0.1;
//Wait until the loop_timer reaches 4000us (250Hz) before starting the next loop
// Print to Serial Monitor
//Serial.print(" | Angle = "); Serial.println(angle_pitch_output);
// Increment the display counter
displaycount = displaycount +1;
//OUTPUT==================================================================
if (displaycount > 100)
{
display.setTextColor(WHITE);
display.clearDisplay();
display.setTextSize(2);
display.setCursor(16,0);
display.print("MPU-6050");
display.setCursor(0,12);
display.print("----------");
display.setCursor(16,25);
display.print("P: "); display.print(angle_pitch_output);
display.setCursor(16,48);
display.print("R: "); display.print(angle_roll_output);
display.display();
// Check Angle for Level LEDs
if (angle_pitch_output < -4.01)
{
tone(buzzer, 1000); delay(500);
}
else if ((angle_pitch_output > -4.00) && (angle_pitch_output < -3.01))
{
digitalWrite(levelLED_neg2, HIGH);
digitalWrite(levelLED_neg1, LOW);
digitalWrite(levelLED_level, LOW);
digitalWrite(levelLED_pos1, LOW);
digitalWrite(levelLED_pos2, LOW);
}
else if ((angle_pitch_output > -2.00) && (angle_pitch_output < -1.01))
{
digitalWrite(levelLED_neg2, LOW);
digitalWrite(levelLED_neg1, HIGH);
digitalWrite(levelLED_level, LOW);
digitalWrite(levelLED_pos1, LOW);
digitalWrite(levelLED_pos2, LOW);
}
else if ((angle_pitch_output < 1.00) && (angle_pitch_output > -1.00))
{
digitalWrite(levelLED_neg2, LOW);
digitalWrite(levelLED_neg1, LOW);
digitalWrite(levelLED_level, HIGH);
digitalWrite(levelLED_pos1, LOW);
digitalWrite(levelLED_pos2, LOW);
}
else if ((angle_pitch_output > 1.01) && (angle_pitch_output < 2.00))
{
digitalWrite(levelLED_neg2, LOW);
digitalWrite(levelLED_neg1, LOW);
digitalWrite(levelLED_level, LOW);
digitalWrite(levelLED_pos1, HIGH);
digitalWrite(levelLED_pos2, LOW);
}
else if ((angle_pitch_output > 3.01) && (angle_pitch_output < 4.00))
{
digitalWrite(levelLED_neg2, LOW);
digitalWrite(levelLED_neg1, LOW);
digitalWrite(levelLED_level, LOW);
digitalWrite(levelLED_pos1, LOW);
digitalWrite(levelLED_pos2, HIGH);
}
else if (angle_pitch_output > 4.01)
{
tone(buzzer, 1000); delay(500);
}
displaycount = 0;
}
//========================================================================
while(micros() - loop_timer < 4000);
//Reset the loop timer
loop_timer = micros();
noTone(buzzer);
}
//FUNCTION=================================================================
void setup_mpu_6050_registers()
{
//Activate the MPU-6050
//Start communicating with the MPU-6050
Wire.beginTransmission(0x68);
//Send the requested starting register
Wire.write(0x6B);
//Set the requested starting register
Wire.write(0x00);
//End the transmission
Wire.endTransmission();
//Configure the accelerometer (+/-8g)
//Start communicating with the MPU-6050
Wire.beginTransmission(0x68);
//Send the requested starting register
Wire.write(0x1C);
//Set the requested starting register
Wire.write(0x10);
//End the transmission
Wire.endTransmission();
//Configure the gyro (500dps full scale)
//Start communicating with the MPU-6050
Wire.beginTransmission(0x68);
//Send the requested starting register
Wire.write(0x1B);
//Set the requested starting register
Wire.write(0x08);
//End the transmission
Wire.endTransmission();
}
//========================================================================
void read_mpu_6050_data()
{
//Read the raw gyro and accelerometer data
//Start communicating with the MPU-6050
Wire.beginTransmission(0x68);
//Send the requested starting register
Wire.write(0x3B);
//End the transmission
Wire.endTransmission();
//Request 14 bytes from the MPU-6050
Wire.requestFrom(0x68,14);
//Wait until all the bytes are received
while(Wire.available() < 14);
//Following statements left shift 8 bits, then bitwise OR.
//Turns two 8-bit values into one 16-bit value
acc_x = Wire.read()<<8|Wire.read();
acc_y = Wire.read()<<8|Wire.read();
acc_z = Wire.read()<<8|Wire.read();
temp = Wire.read()<<8|Wire.read();
gyro_x = Wire.read()<<8|Wire.read();
gyro_y = Wire.read()<<8|Wire.read();
gyro_z = Wire.read()<<8|Wire.read();
}
This code initializes the MPU6050 sensor, reads acceleration data, and calculates the pitch and roll angles, displaying them on the LCD screen.
Testing and Calibration
After building the hardware and uploading the code, it’s time to test and calibrate the device. Ensure that the MPU6050 sensor is properly oriented and level when testing the angles.
Calibration involves adjusting the sensor to minimize any biases in the readings. You may need to fine-tune the code and the physical setup for better accuracy.
Enhancements and Customizations
Once the basic digital spirit level is up and running, you can explore further enhancements:
- Adding a buzzer or LEDs: Alert the user when the device is perfectly level by adding a simple buzzer or LED indicators.
- Bluetooth/Wi-Fi integration: Send data to a mobile device or a cloud platform for remote monitoring.
- Adding more sensors: Use multiple sensors for more precise measurements in different directions.
- 3D printed case: Build a custom enclosure to house your device, making it more portable and durable.
Practical Applications of the Digital Spirit Level
This Arduino-based digital spirit level can be used in various fields, including:
- Construction: Ensure accurate leveling of walls, floors, and equipment.
- DIY projects: Great for hobbyists who need a precise tool for measuring angles.
- Machinery calibration: Use for aligning and calibrating heavy equipment.
FAQs
- How does the MPU6050 sensor work for a spirit level? The MPU6050 uses accelerometers to detect the tilt in three axes. This allows it to measure the angle of inclination, which can then be displayed on a screen.
- Can I use a different sensor instead of the MPU6050? Yes, you can use other accelerometer/gyroscope sensors like the MPU9250, but the MPU6050 is a popular choice due to its affordability and accuracy.
- How accurate is this digital spirit level? The accuracy depends on the calibration and the quality of the MPU6050 sensor. Proper calibration and filtering of the data can significantly improve accuracy.
- Can I integrate this with a mobile app? Yes, you can integrate Bluetooth or Wi-Fi modules like HC-05 or ESP8266 to send the data to a mobile device for remote monitoring.
- Can I make a wireless version of this device? Yes, by using wireless communication modules, you can build a wireless version of the digital spirit level.
Conclusion
The Arduino MPU6050 Digital Spirit Level is a fun and practical project that can be used in many applications. Whether you’re working on DIY projects, construction tasks, or calibrating machinery, this tool provides precise and reliable measurements. The combination of Arduino and MPU6050 gives you a flexible platform for customization and enhancement. Start building your own digital spirit level today and explore the world of Arduino-based projects!
Arduino Projects:
1- Complete Guide for DHT11/DHT22 Humidity and Temperature Sensor With Arduino
2- DHT11 – Temperature and Humidity Sensor
3- DHT22 – Temperature and Humidity Sensor (more accurate than DHT11)
4- BMP180 – Barometric Pressure and Altitude Sensor
5- BMP280 – Barometric Pressure & Temperature Sensor
6- BME280 – Temperature, Humidity, and Pressure Sensor
7- Arduino Flex Sensor Controlled Robot Hand
8- Arduino ECG Heart Rate Monitor AD8232 Demo
9- Arduino NRF24L01 Wireless Joystick Robot Car
10- Arduino Force Sensor Anti-Theft Alarm System
11- Arduino NRF24L01 Transceiver Controlled Relay Light
12- Arduino Rotary Encoder Controlled LEDs: A Complete Guide