Table of Contents
If you’re looking to elevate your airsoft gameplay to the next level, building an Arduino Airsoft Bomb is one of the most creative and immersive projects you can take on. Designed to simulate realistic scenarios like bomb defusal or timed explosions, this DIY prop adds a new layer of intensity and strategy to your matches.
In this guide, you’ll learn how to plan, build, and customize your own Arduino-powered airsoft bomb. We’ll cover everything from components and coding to design aesthetics and troubleshooting. Whether you’re a seasoned maker or a hobbyist just starting out, this guide is built for you.
What Is an Arduino Airsoft Bomb?
An Arduino Airsoft Bomb is a simulated explosive device used in airsoft games to enhance realism. It typically features:
- A countdown timer
- Keypad input for activation or deactivation
- Buzzer and LED indicators for sound and light effects
- Customizable game modes like “Search and Destroy” or “Defuse the Bomb”
For detailed inspiration, check out this Arduino Defusable Bomb Tutorial on Instructables.
Note: These props are purely for recreational use and must be clearly marked as non-lethal devices. Always play responsibly.
Planning Your DIY Project
Before you begin building, it’s important to define the scope and requirements of your project:
- Objective: Choose a specific scenario you want the bomb to simulate
- Budget: Determine your spending cap for parts and tools
- Skills Needed: Basic knowledge of electronics, Arduino programming, and soldering
Get started with free resources from the Arduino Project Hub, which offers beginner-friendly tutorials.
Essential Components and Tools
To build your bomb prop, gather the following:
Core Components
- Arduino Uno or Nano
- 16×2 LCD display
- 4×4 membrane keypad
- Piezo buzzer
- Red/green LEDs
- Push buttons
- Resistors and wires
- 9V battery or power bank
Tools Required
- Soldering iron
- Breadboard
- Wire cutters/strippers
- Screwdriver
- Hot glue gun (for enclosure assembly)
Circuit Design and Hardware Assembly
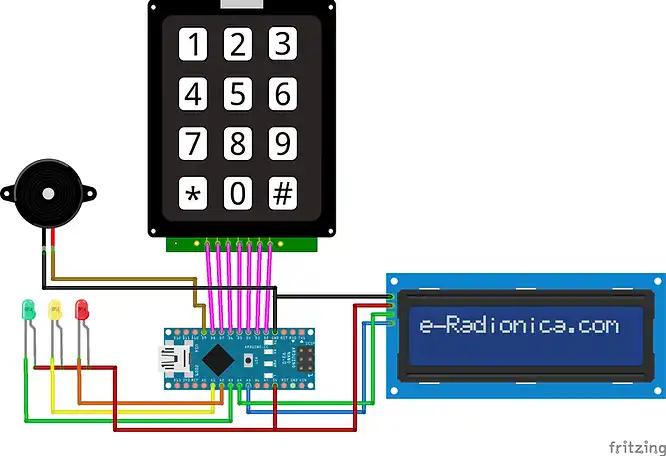
Creating a reliable circuit is key to a functioning device:
- Follow a schematic that includes connections from the Arduino to the keypad, LCD, buzzer, and LEDs
- Use a breadboard for initial testing before soldering
- Secure components with hot glue or screws inside your enclosure
Pro Tip: Label each wire and component to prevent confusion during troubleshooting.
Programming the Arduino
You’ll need to write and upload code that handles the bomb’s core logic:
- Countdown timers with different durations
- Input validation via keypad
- Sound and light alerts on activation and defusal
- Fail-safe reset button
#include <Keypad.h>
#include <Wire.h>
#include <LiquidCrystal_I2C.h>
LiquidCrystal_I2C lcd(0x3F,16,2); //Change the HEX address
int Hours = 00;
int Minutes = 00;
int Seconds = 00;
int trycount = 0;
int keycount = 0;
int i = 0;
int redled = A2;
int yellowled = A1;
int greenled = A3;
int hourstenscode;
int hoursonescode;
int mintenscode;
int minonescode;
int sectenscode;
int seconescode;
long secMillis = 0;
long interval = 1000;
char password[4];
char entered[4];
const byte ROWS = 4;
const byte COLS = 3;
char keys[ROWS][COLS] = {
{'1', '2', '3'},
{'4', '5', '6'},
{'7', '8', '9'},
{'*', '0', '#'}
};
byte rowPins[ROWS] = {8, 7, 6, 5}; //7,2,3,5 for Black 4x3 keypad
byte colPins[COLS] = {4, 3, 2}; //6,8,4 for Black 4x3 Keypad
Keypad keypad = Keypad(makeKeymap(keys), rowPins, colPins, ROWS, COLS);
void setup() {
pinMode(redled, OUTPUT);
pinMode(yellowled, OUTPUT);
pinMode(greenled, OUTPUT);
digitalWrite(redled,HIGH);
digitalWrite(yellowled,HIGH);
digitalWrite(greenled,HIGH);
lcd.begin();
lcd.backlight();
Serial.begin(9600);
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Bomb activated!");
lcd.setCursor(0, 1);
lcd.print("Enter Code:");
while (keycount < 4)
{
lcd.setCursor(keycount + 12, 1);
lcd.blink();
char armcode = keypad.getKey();
armcode == NO_KEY;
if (armcode != NO_KEY)
{
if ((armcode != '*') && (armcode != '#'))
{
lcd.print(armcode);
tone(9, 5000, 100);
password[keycount] = armcode;
keycount++;
}
}
}
if (keycount == 4)
{
delay(500);
lcd.noBlink();
lcd.clear();
lcd.home();
lcd.print("Disarm Code is: ");
lcd.setCursor(6, 1);
lcd.print(password[0]);
lcd.print(password[1]);
lcd.print(password[2]);
lcd.print(password[3]);
delay(3000);
lcd.clear();
}
lcd.setCursor(0, 0);
lcd.print("Timer: HH:MM:SS");
lcd.setCursor(0, 1);
lcd.print("SET: : :");
keycount = 5;
while (keycount == 5)
{
char hourstens = keypad.getKey();
lcd.setCursor(5, 1);
lcd.blink();
if (hourstens >= '0' && hourstens <= '9')
{
hourstenscode = hourstens - '0';
tone(9, 5000, 100);
lcd.print(hourstens);
keycount++;
}
}
while (keycount == 6)
{
char hoursones = keypad.getKey();
lcd.setCursor(6, 1);
lcd.blink();
if (hoursones >= '0' && hoursones <= '9')
{
hoursonescode = hoursones - '0';
tone(9, 5000, 100);
lcd.print(hoursones);
keycount++;
}
}
while (keycount == 7)
{
char mintens = keypad.getKey();
lcd.setCursor(8, 1);
lcd.blink();
if (mintens >= '0' && mintens <= '9')
{
mintenscode = mintens - '0';
tone(9, 5000, 100);
lcd.print(mintens);
keycount++;
}
}
while (keycount == 8)
{
char minones = keypad.getKey();
lcd.setCursor(9, 1);
lcd.blink();
if (minones >= '0' && minones <= '9')
{
minonescode = minones - '0';
tone(9, 5000, 100);
lcd.print(minones);
keycount++;
}
}
while (keycount == 9)
{
char sectens = keypad.getKey();
lcd.setCursor(11, 1);
lcd.blink();
if (sectens >= '0' && sectens <= '9')
{
sectenscode = sectens - '0';
tone(9, 5000, 100);
lcd.print(sectens);
keycount = 10;
}
}
while (keycount == 10)
{
char secones = keypad.getKey();
lcd.setCursor(12, 1);
lcd.blink();
if (secones >= '0' && secones <= '9')
{
seconescode = secones - '0';
tone(9, 5000, 100);
lcd.print(secones);
keycount = 11;
}
}
if (keycount == 11);
{
Hours = (hourstenscode * 10) + hoursonescode;
Minutes = (mintenscode * 10) + minonescode;
Seconds = (sectenscode * 10) + seconescode;
delay(100);
lcd.noBlink();
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Timer set at:");
if (Hours >= 10)
{
lcd.setCursor (7, 1);
lcd.print (Hours);
}
if (Hours < 10)
{
lcd.setCursor (7, 1);
lcd.write ("0");
lcd.setCursor (8, 1);
lcd.print (Hours);
}
lcd.print (":");
if (Minutes >= 10)
{
lcd.setCursor (10, 1);
lcd.print (Minutes);
}
if (Minutes < 10)
{
lcd.setCursor (10, 1);
lcd.write ("0");
lcd.setCursor (11, 1);
lcd.print (Minutes);
}
lcd.print (":");
if (Seconds >= 10)
{
lcd.setCursor (13, 1);
lcd.print (Seconds);
}
if (Seconds < 10)
{
lcd.setCursor (13, 1);
lcd.write ("0");
lcd.setCursor (14, 1);
lcd.print (Seconds);
}
delay(3000);
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Press # to arm");
delay (50);
keycount = 12;
}
while (keycount == 12)
{
char armkey = keypad.getKey();
if (armkey == '#')
{
tone(9, 5000, 100);
delay(50);
tone(9, 0, 100);
delay(50);
tone(9, 5000, 100);
delay(50);
tone(9, 0, 100);
delay(50);
tone(9, 5000, 100);
delay(50);
tone(9, 0, 100);
lcd.clear();
lcd.print ("Bomb Armed!");
lcd.setCursor(0, 1);
lcd.print("Countdown start");
delay(3000);
lcd.clear();
keycount = 0;
}
}
}
void loop()
{
timer();
char disarmcode = keypad.getKey();
if (disarmcode == '*')
{
tone(9, 5000, 100);
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Code: ");
while (keycount < 4)
{
timer();
char disarmcode = keypad.getKey();
if (disarmcode == '#')
{
tone(9, 5000, 100);
keycount = 0;
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Code: ");
}
else if (disarmcode != NO_KEY)
{
lcd.setCursor(keycount + 7, 0);
lcd.blink();
lcd.print(disarmcode);
entered[keycount] = disarmcode;
keycount++;
tone(9, 5000, 100);
delay(100);
lcd.noBlink();
lcd.setCursor(keycount + 6, 0);
lcd.print("*");
lcd.setCursor(keycount + 7, 0);
lcd.blink();
}
}
if (keycount == 4)
{
if (entered[0] == password[0] && entered[1] == password[1] && entered[2] == password[2] && entered[3] == password[3])
{
lcd.noBlink();
lcd.clear();
lcd.home();
lcd.print("Bomb Defused!");
lcd.setCursor(0, 1);
lcd.print("Well Done!");
keycount = 0;
digitalWrite(greenled, LOW);
delay(15000);
lcd.clear();
lcd.setCursor(0, 1);
lcd.print("Reset the Bomb");
delay(1000000);
}
else
{
lcd.noBlink();
lcd.clear();
lcd.home();
lcd.print("Wrong Password!");
trycount++;
if (Hours > 0)
{
Hours = Hours / 2;
}
if (Minutes > 0)
{
Minutes = Minutes / 2;
}
if (Seconds > 0)
{
Seconds = Seconds / 2;
}
if (trycount == 2)
{
interval = interval / 10;
}
if (trycount == 3)
{
Minutes = Minutes - 59;
Hours = Hours - 59;
Seconds = Seconds - 59;
}
delay(1000);
keycount = 0;
}
}
}
}
void timer()
{
Serial.print(Seconds);
Serial.println();
if (Hours <= 0)
{
if ( Minutes < 0 )
{
lcd.noBlink();
lcd.clear();
lcd.home();
lcd.print("The Bomb Has ");
lcd.setCursor (0, 1);
lcd.print("Exploded!");
while (Minutes < 0)
{
digitalWrite(redled, LOW);
tone(9, 7000, 100);
delay(100);
digitalWrite(redled, HIGH);
tone(9, 7000, 100);
delay(100);
digitalWrite(yellowled, LOW);
tone(9, 7000, 100);
delay(100);
digitalWrite(yellowled, HIGH);
tone(9, 7000, 100);
delay(100);
digitalWrite(greenled, LOW);
tone(9, 7000, 100);
delay(100);
digitalWrite(greenled, HIGH);
tone(9, 7000, 100);
delay(100);
}
}
}
lcd.setCursor (0, 1);
lcd.print ("Timer:");
if (Hours >= 10)
{
lcd.setCursor (7, 1);
lcd.print (Hours);
}
if (Hours < 10)
{
lcd.setCursor (7, 1);
lcd.write ("0");
lcd.setCursor (8, 1);
lcd.print (Hours);
}
lcd.print (":");
if (Minutes >= 10)
{
lcd.setCursor (10, 1);
lcd.print (Minutes);
}
if (Minutes < 10)
{
lcd.setCursor (10, 1);
lcd.write ("0");
lcd.setCursor (11, 1);
lcd.print (Minutes);
}
lcd.print (":");
if (Seconds >= 10)
{
lcd.setCursor (13, 1);
lcd.print (Seconds);
}
if (Seconds < 10)
{
lcd.setCursor (13, 1);
lcd.write ("0");
lcd.setCursor (14, 1);
lcd.print (Seconds);
}
if (Hours < 0)
{
Hours = 0;
}
if (Minutes < 0)
{
Hours --;
Minutes = 59;
}
if (Seconds < 1)
{
Minutes --;
Seconds = 59;
}
if (Seconds > 0)
{
unsigned long currentMillis = millis();
if (currentMillis - secMillis > interval)
{
tone(9, 7000, 50);
secMillis = currentMillis;
Seconds --;
digitalWrite(yellowled, LOW);
delay(10);
digitalWrite(yellowled, HIGH);
delay(10);
}
}
}
Use the Arduino IDE to compile and upload your code. Libraries like Keypad.h
and LiquidCrystal.h
are essential.
Adding Game Modes and Features
To enhance realism, consider these customizations:
- Game Modes:
- Search and Destroy: Arm and disarm sequences
- Timed Explosion: Detonates if not disarmed in time
- Code Entry: Only specific codes defuse the bomb
- Feedback Options:
- Vibrating motor for tactile alerts
- RGB LEDs for multiple status indicators
- Sound effects like ticking or alarms
Designing the Enclosure
Your bomb’s visual appeal affects its realism. Design tips:
- Use a durable plastic or wood box
- Cut openings for the display and keypad
- Paint the case black or camouflage green
- Use printed labels or stencils for functions like “ARM” and “DEFUSE”
Testing and Troubleshooting
After assembly, test your prop in a controlled environment:
- Check all connections and sensors
- Test each game mode multiple times
- Replace weak batteries
- Watch for faulty solder joints or unresponsive buttons
FAQs
Q: How do I reset the bomb after each game?
A reset button can be programmed to restart the timer and state machine.
Q: Can I use a different Arduino model?
Yes, models like the Arduino Nano or Mega are also compatible.
Q: How can I extend the battery life?
Use a rechargeable power bank or larger capacity battery and optimize your code to sleep between cycles.
Q: Can I add wireless control?
Yes, modules like NRF24L01 or Bluetooth HC-05 can enable remote control.
Q: Is it safe for use in public areas?
Always mark your prop clearly and use it only in designated airsoft fields.
Conclusion
Building an Arduino Airsoft Bomb is not only a fun and rewarding project but also an excellent way to enhance your airsoft games with an added layer of strategy and excitement. By using the right components like an Arduino board, keypad, buzzer, and LEDs, combined with a bit of creative coding, you can create a realistic bomb prop that functions just like the ones seen in action-packed video games and movies. Whether you’re designing a countdown timer, adding game modes, or fine-tuning your enclosure, each step offers opportunities for innovation.
This project encourages a mix of practical skills and creative problem-solving, perfect for makers, hobbyists, and airsoft enthusiasts alike. Once assembled and programmed, the bomb prop can become a central element of your airsoft scenarios, increasing immersion and competitiveness for everyone involved.
Additionally, once you’ve built your device, don’t hesitate to tweak and test it for new features or enhancements. Consider integrating wireless controls, more complex game modes, or even multiplayer support for larger events. Whatever you choose, your Arduino Airsoft Bomb is sure to add thrilling dynamics to your next airsoft match—taking your gameplay to a whole new level of excitement!
Arduino Projects:
1- Complete Guide for DHT11/DHT22 Humidity and Temperature Sensor With Arduino
2- DHT11 – Temperature and Humidity Sensor
3- DHT22 – Temperature and Humidity Sensor (more accurate than DHT11)
4- BMP180 – Barometric Pressure and Altitude Sensor
5- BMP280 – Barometric Pressure & Temperature Sensor
6- BME280 – Temperature, Humidity, and Pressure Sensor
7- Arduino Flex Sensor Controlled Robot Hand
8- Arduino ECG Heart Rate Monitor AD8232 Demo
9- Arduino NRF24L01 Wireless Joystick Robot Car
10- Arduino Force Sensor Anti-Theft Alarm System
11- Arduino NRF24L01 Transceiver Controlled Relay Light
12- Arduino Rotary Encoder Controlled LEDs: A Complete Guide