Table of Contents
1. Introduction
The Arduino Tetris Game using the Max7219 8×8 Matrix Display is an exciting project for DIY enthusiasts and game developers. This project involves programming an Arduino to render the classic Tetris game on an LED matrix display while using a joystick module for control.
Understanding how to integrate Arduino with the Max7219 LED matrix is essential for building this game. The Max7219 module simplifies LED control, making it perfect for dynamic visual applications. Additionally, the project is an excellent way to learn about SPI communication, game logic, and real-time inputs.
For a deeper understanding of how the Max7219 works, refer to this Max7219 LED Driver Guide.
2. Components Required
To build this Arduino Tetris Game, you will need the following components:
- Arduino Uno (or any compatible board)
- Max7219 8×8 LED Matrix Display
- Joystick Module
- Push Buttons (Optional for additional controls)
- Resistors (10kΩ)
- Breadboard and Jumper Wires
- Power Supply (9V battery or USB power)
For more details on joystick modules, check out this Joystick Module Tutorial.
3. Circuit Diagram & Wiring
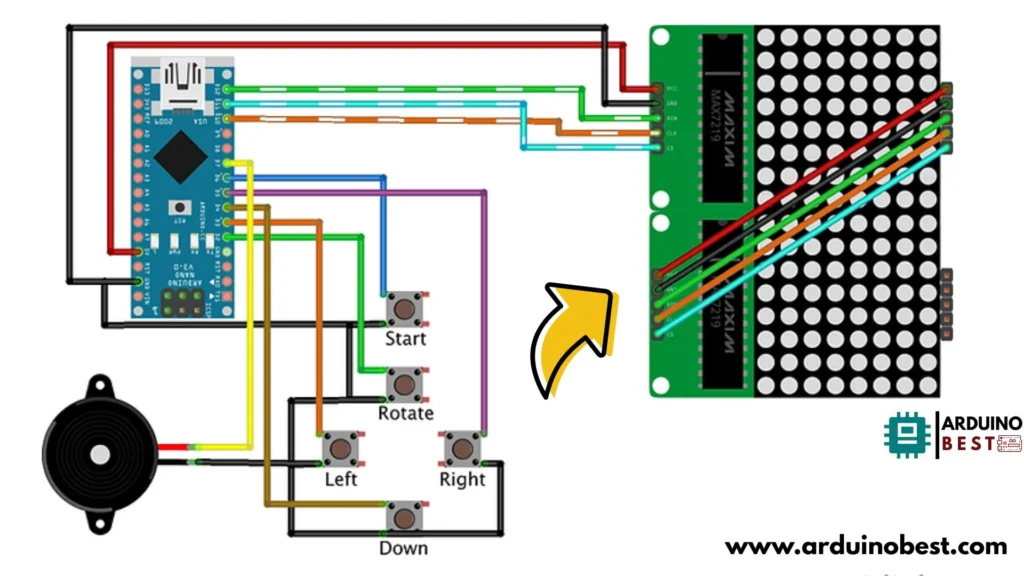
3.1 Connecting the Max7219 LED Matrix to Arduino
Max7219 Pins | Arduino Pins |
---|---|
VCC | 5V |
GND | GND |
DIN | Digital Pin 11 |
CS | Digital Pin 10 |
CLK | Digital Pin 13 |
3.2 Connecting the Joystick Module to Arduino
Joystick Pins | Arduino Pins |
---|---|
VCC | 5V |
GND | GND |
VRX | Analog Pin A0 |
VRY | Analog Pin A1 |
SW | Digital Pin 2 |
3.3 Powering the Circuit
- Use a 9V battery or connect through USB power.
- Ensure a common ground connection between all components.
4. Understanding the Game Logic
4.1 Basics of Tetris
- Tetrominoes: The game consists of different shapes (tetrominoes) that must be arranged efficiently.
- Game Grid: The LED matrix acts as a 10×20 virtual game grid.
- Scoring System: Players gain points by clearing full rows.
- Game Over Condition: The game ends when the blocks stack to the top.
4.2 Movement and Rotation
- The joystick moves blocks left or right.
- Pressing the joystick button rotates the block.
- Blocks fall automatically, and speed increases over time.
5. Writing the Arduino Code
5.1 Installing Required Libraries
Before writing the code, install the following libraries:
#include <LedControl.h>
#include <EEPROM.h>
5.2 Defining Pins and Variables
#define DATA_IN 11
#define CS 10
#define CLK 13
LedControl lc = LedControl(DATA_IN, CLK, CS, 1);
int joystickX = A0;
int joystickY = A1;
int buttonPin = 2;
5.3 Initializing the LED Matrix
void setup() {
pinMode(joystickX, INPUT);
pinMode(joystickY, INPUT);
pinMode(buttonPin, INPUT_PULLUP);
lc.shutdown(0, false);
lc.setIntensity(0, 5);
lc.clearDisplay(0);
}
5.4 Game Loop and Controls
#include <LedControl.h>
LedControl lc = LedControl(12, 11, 10, 2); // (dataPin, clockPin, csPin, totalDevices)
int lc0[] = {0, 0, 0, 0, 0, 0, 0, 0};
int lc1[] = {0, 0, 0, 0, 0, 0, 0, 0};
long active[] = {0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0};
long screen[] = {0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0};
int tmpCol = 0;
int figura = 0;
int figuraNext = 0;
int fromLeft = 0;
int fromRight = 0;
int angle = 0;
int colCheck = 0;
int moveCheck = 0;
int score = 0;
int started = 0;
int lcRows = 16;
int lcCols = 8;
int allLines = 0;
int currLines = 0;
int brickDelay = 0;
int defDelay = 500;
int level = 0;
boolean sound = true;
//Pinos
int rotate_button = 2;
int left_button = 3;
int down_button = 4;
int right_button = 5;
int start_button = 6;
int speaker_pin = 7;
int sound_button = 8;
byte X[8] =
{
0b00000,
0b10001,
0b01010,
0b00100,
0b01010,
0b10001,
0b00000,
0b00000
};
byte O[8] =
{
0b00000,
0b11111,
0b11111,
0b11111,
0b11111,
0b11111,
0b00000,
0b00000
};
byte L[8] =
{
0b11000,
0b11000,
0b11000,
0b11000,
0b11000,
0b11111,
0b11111,
0b00000
};
byte J[8] =
{
0b00011,
0b00011,
0b00011,
0b00011,
0b00011,
0b11111,
0b11111,
0b00000
};
byte T[8] =
{
0b00000,
0b00000,
0b11111,
0b11111,
0b01110,
0b01110,
0b00000,
0b00000
};
byte I[8] =
{
0b01100,
0b01100,
0b01100,
0b01100,
0b01100,
0b01100,
0b01100,
0b00000
};
byte Z[8] =
{
0b00000,
0b00000,
0b11110,
0b11110,
0b01111,
0b01111,
0b00000,
0b00000
};
byte S[8] =
{
0b00000,
0b00000,
0b01111,
0b01111,
0b11110,
0b11110,
0b00000,
0b00000
};
//Nuty
int length = 99;
char notes[] = "EbCDCbaaCEDCbbCDECaa DFAGFEECEDCbbCDECaa EbCDCbaaCEDCbbCDECaa DFAGFEECEDCbbCDECaa ECDbCab ECDbCEAJ ";
int beats[] = // Som
{
2, 1, 1, 2, 1, 1, 2, 1, 1, 2, 1, 1, 2, 1, 1, 2, 2, 2, 2, 4, 2,
2, 1, 2, 1, 1, 2, 1, 1, 2, 1, 1, 2, 1, 1, 2, 2, 2, 2, 4, 1,
2, 1, 1, 2, 1, 1, 2, 1, 1, 2, 1, 1, 2, 1, 1, 2, 2, 2, 2, 4, 2,
2, 1, 2, 1, 1, 2, 1, 1, 2, 1, 1, 2, 1, 1, 2, 2, 2, 2, 4, 1,
5, 5, 5, 5, 5, 5, 7, 2, 5, 5, 5, 5, 2, 2, 5, 5, 3
};
int tempo = 128; // Tempo
void playTone(int tone, int duration) {
for (long i = 0; i < duration * 1000L; i += tone * 2) {
digitalWrite(speaker_pin, HIGH);
delayMicroseconds(tone);
digitalWrite(speaker_pin, LOW);
delayMicroseconds(tone);
}
}
void playNote(char note, int duration) {
char names[] = { 'c', 'd', 'e', 'f', 'g', 'a', 'b', 'C' , 'D', 'E', 'F', 'G', 'J', 'A', 'B'};
int tones[] = { 1915, 1700, 1519, 1432, 1275, 1136, 1014, 956, 850, 760, 716, 637, 603, 568, 507 };
for (int i = 0; i < 14; i++) {
if (names[i] == note) {
playTone(tones[i], duration);
}
}
}
void updateColumn(int colnum)
{
lc0[colnum] = active[colnum] >> (lcRows / 2);
lc1[colnum] = active[colnum];
lc.setColumn(0,colnum,(screen[colnum] >> (lcRows / 2)) | lc0[colnum]);
lc.setColumn(1,colnum,screen[colnum] | lc1[colnum]);
}
void buttonDelay(int bdelay)
{
if(brickDelay > bdelay)
{
brickDelay -= bdelay;
}
delay(bdelay);
}
void splashScreen()
{
int up[] =
{
B11101110, // o o o o o o
B01001000, // o o
B01001100, // o o o
B01001000, // o o
B01001110, // o o o o
B00000000, //
B11101110, // o o o o o o
B01001010 // o o o
};
int down[] =
{
B01001100, // o o o
B01001010, // o o o
B01001001, // o o o
B00000000, //
B01000111, // o o o o
B01000100, // o o
B01000010, // o o
B01001110 // o o o o
};
for(int rownum = 0; rownum < 8; rownum++)
{
lc.setRow(0,rownum,up[rownum]);
lc.setRow(1,rownum,down[rownum]);
}
}
void setup() {
pinMode(speaker_pin, OUTPUT);
pinMode(rotate_button,INPUT_PULLUP);
pinMode(down_button, INPUT_PULLUP);
pinMode(right_button, INPUT_PULLUP);
pinMode(left_button, INPUT_PULLUP);
pinMode(start_button, INPUT_PULLUP);
pinMode(sound_button, INPUT_PULLUP);
lc.shutdown(0,false);
lc.shutdown(1,false);
lc.setIntensity(0,5);
lc.setIntensity(1,5);
lc.clearDisplay(0);
lc.clearDisplay(1);
Serial.begin(9600);
randomSeed(analogRead(0));
}
//LOOP
void loop()
{
if(started == 0)
{
splashScreen();
for (int i = 0; i < length; i++)
{
if(digitalRead(sound_button) == LOW)
{
sound =! sound;
delay(300);
}
if(digitalRead(start_button) == LOW)
{
started = 1;
break;
}
if (notes[i] == ' ')
{
delay(beats[i] * tempo); //Pausa
}
else
{
if (sound == 1){
playNote(notes[i], beats[i] * tempo);
}
else
{
digitalWrite(speaker_pin,LOW);
}
}
delay(tempo / 2);
}
}
else
{
lc.clearDisplay(0);
lc.clearDisplay(1);
memset(lc0, 0, sizeof(lc0));
memset(lc1, 0, sizeof(lc1));
memset(active, 0, sizeof(active));
memset(screen, 0, sizeof(screen));
tmpCol = 0;
while(started == 1)
{
if(allLines < 100)
{
level = 0; //Level 0
}
else if(allLines < 200)
{
level = 1; //Level 1
}
else if(allLines < 300)
{
level = 2; //Level 2
}
else if(allLines < 400)
{
level = 3; //Level 3
}
else if(allLines < 500)
{
level = 4; //Level 4
}
else
{
level = 5; //Level 5
}
defDelay = (5 - level) * 100;
brickDelay = defDelay;
if(figura == 0)
{
figura = random(1,8);
}
else
{
figura = figuraNext;
}
figuraNext = random(1,8);
angle = 0;
switch(figura)
{
case 1:
//"O"
active[3] = 131072 + 65536;
active[4] = 131072 + 65536;
fromLeft = 3;
fromRight = 3;
break;
case 2:
//"L"
active[3] = 262144 + 131072 + 65536;
active[4] = 65536;
fromLeft = 3;
fromRight = 3;
break;
case 3:
//"J"
active[3] = 65536;
active[4] = 262144 + 131072 + 65536;
fromLeft = 3;
fromRight = 3;
break;
case 4:
//"T"
active[2] = 131072;
active[3] = 131072 + 65536;
active[4] = 131072;
fromLeft = 2;
fromRight = 3;
break;
case 5:
//"I"
active[3] = 524288 + 262144 + 131072 + 65536;
fromLeft = 3;
fromRight = 4;
break;
case 6:
//"Z"
active[2] = 131072;
active[3] = 131072 + 65536;
active[4] = 65536;
fromLeft = 2;
fromRight = 3;
break;
case 7:
//"S"
active[2] = 65536;
active[3] = 131072 + 65536;
active[4] = 131072;
fromLeft = 2;
fromRight = 3;
break;
}
for(int krok = 0; krok < lcRows + 1; krok++)
{
colCheck = 0;
for(int i = 0; i < (lcCols / 2); i++)
{
if((digitalRead(left_button) == LOW) && (fromLeft > 0))
{
moveCheck = 0;
for(int colnum = fromLeft; colnum < (lcCols - fromRight); colnum++)
{
if((active[colnum] & screen[colnum - 1]) == 0)
{
moveCheck++;
}
}
if(moveCheck == (lcCols - fromLeft - fromRight))
{
for(int colnum = (fromLeft - 1); colnum < (lcCols - fromRight); colnum++)
{
if(colnum < (lcCols - 1))
{
active[colnum] = active[colnum+1];
}
else
{
active[colnum] = 0;
}
updateColumn(colnum);
}
fromLeft--;
fromRight++;
playNote('E', 10);
buttonDelay(200);
}
}
}
for(int i = 0; i < (lcCols / 2); i++)
{
if((digitalRead(right_button) == LOW) && (fromRight > 0))
{
moveCheck = 0;
for(int colnum = fromLeft; colnum < (lcCols - fromRight); colnum++)
{
if((active[colnum] & screen[colnum + 1]) == 0)
{
moveCheck++;
}
}
if(moveCheck == (lcCols - fromLeft - fromRight))
{
for(int colnum = (lcCols - fromRight); colnum > (fromLeft - 1); colnum--)
{
if(colnum > 0)
{
active[colnum] = active[colnum-1];
}
else
{
active[colnum] = 0;
}
updateColumn(colnum);
}
fromLeft++;
fromRight--;
playNote('E', 10);
buttonDelay(200);
}
}
}
if(digitalRead(down_button) == LOW)
{
brickDelay = 0;
playNote('b', 10);
}
else
{
brickDelay = defDelay;
}
for(int i = 0; i < (lcCols / 2); i++)
{
if(digitalRead(rotate_button) == LOW)
{
switch(figura)
{
case 1:
//"O"
break;
case 2:
//"L"
switch(angle)
{
case 0:
// . o . . . .
// . o . ---> o o o
// . o o o . .
if((fromLeft > 0)
&& (((active[fromLeft + 1] | (active[fromLeft + 1] << 1)) & screen[fromLeft - 1]) == 0))
{
active[fromLeft - 1] = (active[fromLeft + 1] | (active[fromLeft + 1] << 1));
updateColumn(fromLeft - 1);
active[fromLeft] = (active[fromLeft + 1] << 1);
updateColumn(fromLeft);
active[fromLeft + 1] = (active[fromLeft + 1] << 1);
updateColumn(fromLeft + 1);
fromLeft--;
angle = 1;
}
break;
case 1:
// . . . o o .
// o o o ---> . o .
// o . . . o .
if((((active[fromLeft + 2] << 1) & screen[fromLeft]) == 0)
&& ((((active[fromLeft + 1] << 1) | (active[fromLeft + 1] >> 1)) & screen[fromLeft + 1]) == 0))
{
active[fromLeft] = (active[fromLeft + 2] << 1);
updateColumn(fromLeft);
active[fromLeft + 1] = active[fromLeft + 1] | (active[fromLeft + 1] << 1) | (active[fromLeft + 1] >> 1);
updateColumn(fromLeft + 1);
active[fromLeft + 2] = 0;
updateColumn(fromLeft + 2);
fromRight++;
angle = 2;
}
break;
case 2:
// o o . . . o
// . o . ---> o o o
// . o . . . .
if((fromRight > 0)
&& (((active[fromLeft] >> 1) & screen[fromLeft]) == 0)
&& ((((active[fromLeft + 1] << 1) & active[fromLeft + 1]) & screen[fromLeft + 1]) == 0))
{
active[fromLeft] = (active[fromLeft] >> 1);
updateColumn(fromLeft);
active[fromLeft + 1] = active[fromLeft];
updateColumn(fromLeft + 1);
active[fromLeft + 2] = ((active[fromLeft + 1] << 1) | active[fromLeft + 1]);
updateColumn(fromLeft + 2);
fromRight--;
krok--;
angle = 3;
}
break;
case 3:
// . . o . o .
// o o o ---> . o .
// . . . . o o
if(((((active[fromLeft] << 1) | (active[fromLeft] >> 1)) & screen[fromLeft + 1]) == 0)
&& (((active[fromLeft] >> 1) & screen[fromLeft + 2]) == 0)
&& (krok < lcRows))
{
active[fromLeft] = 0;
updateColumn(fromLeft);
active[fromLeft + 1] = (active[fromLeft + 2] | (active[fromLeft + 2] >> 1));
updateColumn(fromLeft + 1);
active[fromLeft + 2] = ((active[fromLeft + 2] >> 1) & (active[fromLeft + 2] >> 2));
updateColumn(fromLeft + 2);
fromLeft++;
krok++;
angle = 0;
}
break;
}
break;
case 3:
//"J"
switch(angle)
{
case 0:
// . o . o . .
// . o . ---> o o o
// o o . . . .
if((fromRight > 0)
&& ((((active[fromLeft] << 2) | (active[fromLeft] << 1)) & screen[fromLeft]) == 0)
&& (((active[fromLeft] << 1) & screen[fromLeft + 2]) == 0))
{
active[fromLeft] = ((active[fromLeft] << 2) | (active[fromLeft] << 1));
updateColumn(fromLeft);
active[fromLeft + 1] = ((active[fromLeft + 1] << 1) & (active[fromLeft + 1] >> 1));
updateColumn(fromLeft + 1);
active[fromLeft + 2] = active[fromLeft + 1];
updateColumn(fromLeft + 2);
fromRight--;
krok--;
angle = 1;
}
break;
case 1:
// o . . . o o
// o o o ---> . o .
// . . . . o .
if((krok < lcRows)
&& ((((active[fromLeft + 1] << 1) | (active[fromLeft + 1] >> 1)) & screen[fromLeft + 1]) == 0)
&& (((active[fromLeft + 2] << 1) & screen[fromLeft + 2]) == 0))
{
active[fromLeft] = 0;
updateColumn(fromLeft);
active[fromLeft + 1] = (active[fromLeft + 1] | (active[fromLeft + 1] << 1) | (active[fromLeft + 1] >> 1));
updateColumn(fromLeft + 1);
active[fromLeft + 2] = (active[fromLeft + 2] << 1);
updateColumn(fromLeft + 2);
fromLeft++;
krok++;
angle = 2;
}
break;
case 2:
// . o o . . .
// . o . ---> o o o
// . o . . . o
if((fromLeft > 0)
&& (((active[fromLeft + 1] >> 1) & screen[fromLeft - 1]) == 0)
&& ((((active[fromLeft + 1] >> 1) | (active[fromLeft + 1] >> 2)) & screen[fromLeft + 1]) == 0))
{
active[fromLeft - 1] = (active[fromLeft + 1] >> 1);
updateColumn(fromLeft - 1);
active[fromLeft] = active[fromLeft - 1];
updateColumn(fromLeft);
active[fromLeft + 1] = (active[fromLeft] | (active[fromLeft + 1] >> 2));
updateColumn(fromLeft + 1);
fromLeft--;
angle = 3;
}
break;
case 3:
// . . . . o .
// o o o ---> . o .
// . . o o o .
if((((active[fromLeft] >> 1) & screen[fromLeft]) == 0)
&& ((((active[fromLeft] << 1) | (active[fromLeft >> 1])) & screen[fromLeft + 1]) == 0))
{
active[fromLeft] = (active[fromLeft] >> 1);
updateColumn(fromLeft);
active[fromLeft + 1] = ((active[fromLeft + 1] << 1) | active[fromLeft + 2]);
updateColumn(fromLeft + 1);
active[fromLeft + 2] = 0;
updateColumn(fromLeft + 2);
fromRight++;
angle = 0;
}
break;
}
break;
case 4:
//"T"
switch(angle)
{
case 0:
// . . . . o .
// o o o ---> o o .
// . o . . o .
if(((active[fromLeft + 1] << 1) & screen[fromLeft + 1]) == 0)
{
//active[fromLeft]
active[fromLeft + 1] = active[fromLeft + 1] | (active[fromLeft + 1] << 1);
updateColumn(fromLeft + 1);
active[fromLeft + 2] = 0;
updateColumn(fromLeft + 2);
fromRight++;
angle = 1;
}
break;
case 1:
// . o . . o .
// o o . ---> o o o
// . o . . . .
if((fromRight > 0)
&& ((active[fromLeft] & screen[fromLeft + 2])== 0))
{
//active[fromLeft]
active[fromLeft + 1] = active[fromLeft + 1] & (active[fromLeft + 1] << 1);
updateColumn(fromLeft + 1);
active[fromLeft + 2] = active[fromLeft];
updateColumn(fromLeft + 2);
fromRight--;
krok--;
angle = 2;
}
break;
case 2:
// . o . . o .
// o o o ---> . o o
// . . . . o .
if((((active[fromLeft + 1] >> 1) & screen[fromLeft + 1]) == 0)
&& (krok < lcRows))
{
active[fromLeft] = 0;
updateColumn(fromLeft);
active[fromLeft + 1] = active[fromLeft + 1] | (active[fromLeft + 1] >> 1);
updateColumn(fromLeft + 1);
//active[fromLeft + 2]
fromLeft++;
krok++;
angle = 3;
}
break;
case 3:
if((fromLeft > 0)
&& ((active[fromLeft + 1] & screen[fromLeft - 1]) == 0))
{
active[fromLeft - 1] = active[fromLeft + 1];
updateColumn(fromLeft - 1);
active[fromLeft] = active[fromLeft] & (active[fromLeft] >> 1);
updateColumn(fromLeft);
fromLeft--;
angle = 0;
}
break;
}
break;
case 5:
//"I"
switch(angle)
{
case 0:
// . o . . . . . .
// . o . . ---> o o o o
// . o . . . . . .
// . o . . . . . .
if((fromLeft > 0)
&& (fromRight > 1)
&& ((((((active[fromLeft] >> 1) & (active[fromLeft] << 2)) & screen[fromLeft - 1]) & screen[fromLeft + 1]) & screen[fromLeft + 2]) == 0))
{
active[fromLeft - 1] = ((active[fromLeft] >> 1) & (active[fromLeft] << 2));
updateColumn(fromLeft - 1);
active[fromLeft] = active[fromLeft - 1];
updateColumn(fromLeft);
active[fromLeft + 1] = active[fromLeft];
updateColumn(fromLeft + 1);
active[fromLeft + 2] = active[fromLeft];
updateColumn(fromLeft + 2);
fromLeft--;
fromRight -= 2;
krok -= 2;
angle = 1;
}
break;
case 1:
// . . . . . . o .
// o o o o ---> . . o .
// . . . . . . o .
// . . . . . . o .
if((krok < (lcRows - 1))
&& (((active[fromLeft] << 1) | (active[fromLeft] >> 1) | (active[fromLeft] >> 2)) & screen[fromLeft + 2]) == 0)
{
active[fromLeft] = 0;
updateColumn(fromLeft);
active[fromLeft + 1] = 0;
updateColumn(fromLeft + 1);
active[fromLeft + 2] = (active[fromLeft + 2] | (active[fromLeft + 2] << 1) | (active[fromLeft + 2] >> 1) | (active[fromLeft + 2] >> 2));
updateColumn(fromLeft + 2);
active[fromLeft + 3] = 0;
updateColumn(fromLeft + 3);
fromLeft += 2;
fromRight++;
krok += 2;
angle = 2;
}
break;
case 2:
// . . o . . . . .
// . . o . ---> . . . .
// . . o . o o o o
// . . o . . . . .
if((fromLeft > 1)
&& (fromRight > 0)
&& ((((((active[fromLeft] << 1) & (active[fromLeft] >> 2)) & screen[fromLeft - 2]) & screen[fromLeft - 1]) & screen[fromLeft + 1]) == 0))
{
active[fromLeft - 2] = ((active[fromLeft] << 1) & (active[fromLeft] >> 2));
updateColumn(fromLeft - 2);
active[fromLeft - 1] = active[fromLeft - 2];
updateColumn(fromLeft - 1);
active[fromLeft] = active[fromLeft - 1];
updateColumn(fromLeft);
active[fromLeft + 1] = active[fromLeft];
updateColumn(fromLeft + 1);
fromLeft -= 2;
fromRight--;
krok--;
angle = 3;
}
break;
case 3:
// . . . . . o . .
// . . . . ---> . o . .
// o o o o . o . .
// . . . . . o . .
if((krok < (lcRows))
&& (((active[fromLeft] >> 1) | (active[fromLeft] << 1) | (active[fromLeft] << 2)) & screen[fromLeft + 1]) == 0)
{
active[fromLeft] = 0;
updateColumn(fromLeft);
active[fromLeft + 1] = (active[fromLeft + 1] | (active[fromLeft + 1] >> 1) | (active[fromLeft + 1] << 1) | (active[fromLeft + 1] << 2));
updateColumn(fromLeft + 1);
active[fromLeft + 2] = 0;
updateColumn(fromLeft + 2);
active[fromLeft + 3] = 0;
updateColumn(fromLeft + 3);
fromLeft++;
fromRight += 2;
krok++;
angle = 0;
}
break;
}
break;
case 6:
//"Z"
switch(angle)
{
case 0:
// . . . . o .
// o o . ---> o o .
// . o o o . .
if(((active[fromLeft + 1] & screen[fromLeft]) == 0)
&& (((active[fromLeft + 1] << 1) & screen[fromLeft + 1]) == 0))
{
active[fromLeft] = active[fromLeft + 1];
updateColumn(fromLeft);
active[fromLeft + 1] = (active[fromLeft + 1] << 1);
updateColumn(fromLeft + 1);
active[fromLeft + 2] = 0;
updateColumn(fromLeft + 2);
fromRight++;
angle = 1;
}
break;
case 1:
// . o . o o .
// o o . ---> . o o
// o . . . . .
if((fromRight > 0)
&& ((((active[fromLeft] << 2) & (active[fromLeft] << 1)) & screen[fromLeft]) == 0)
&& (((active[fromLeft] & active[fromLeft + 1]) & screen[fromLeft + 2]) == 0))
{
active[fromLeft] = ((active[fromLeft] << 2) & (active[fromLeft] << 1));
updateColumn(fromLeft);
//active[fromLeft + 1]
active[fromLeft + 2] = (active[fromLeft] >> 1);
updateColumn(fromLeft + 2);
fromRight--;
krok--;
angle = 2;
}
break;
case 2:
// o o . . . o
// . o o ---> . o o
// . . . . o .
if((krok < lcRows)
&& (((active[fromLeft + 1] >> 1) & screen[fromLeft + 1]) == 0)
&& (((active[fromLeft + 2] << 1) & screen[fromLeft + 2]) == 0))
{
active[fromLeft] = 0;
updateColumn(fromLeft);
active[fromLeft + 1] = (active[fromLeft + 1] >> 1);
updateColumn(fromLeft + 1);
active[fromLeft + 2] = (active[fromLeft + 2] | (active[fromLeft + 2] << 1));
updateColumn(fromLeft + 2);
fromLeft++;
krok++;
angle = 3;
}
break;
case 3:
// . . o . . .
// . o o ---> o o .
// . o . . o o
if((fromLeft > 0)
&& (((active[fromLeft] & active[fromLeft + 1]) & screen[fromLeft - 1]) == 0)
&& (((active[fromLeft + 1] >> 1) & screen[fromLeft + 1]) == 0))
{
active[fromLeft - 1] = (active[fromLeft] & active[fromLeft + 1]);
updateColumn(fromLeft - 1);
//active[fromLeft]
active[fromLeft + 1] = (active[fromLeft - 1] >> 1);
updateColumn(fromLeft + 1);
fromLeft--;
angle = 0;
}
break;
}
break;
case 7:
//"S"
switch(angle)
{
case 0:
// . . . o . .
// . o o ---> o o .
// o o . . o .
if(((active[fromLeft + 1] << 1) & screen[fromLeft]) == 0)
{
active[fromLeft] = (active[fromLeft + 1] << 1);
updateColumn(fromLeft);
//active[fromLeft + 1]
active[fromLeft + 2] = 0;
updateColumn(fromLeft + 2);
fromRight++;
angle = 1;
}
break;
case 1:
// o . . . o o
// o o . ---> o o .
// . o . . . .
if((fromRight > 0)
&& (((active[fromLeft + 1] << 1) & screen[fromLeft + 1]) == 0)
&& (((active[fromLeft] & (active[fromLeft] << 1)) & screen[fromLeft + 2]) == 0))
{
active[fromLeft] = (active[fromLeft] & active[fromLeft + 1]);
updateColumn(fromLeft);
active[fromLeft + 1] = (active[fromLeft + 1] << 1);
updateColumn(fromLeft + 1);
active[fromLeft + 2] = (active[fromLeft] << 1);
updateColumn(fromLeft + 2);
fromRight--;
krok--;
angle = 2;
}
break;
case 2:
// . o o . o .
// o o . ---> . o o
// . . . . . o
if((krok < lcRows)
&& (((active[fromLeft + 1] >> 1) & screen[fromLeft + 2]) == 0))
{
active[fromLeft] = 0;
updateColumn(fromLeft);
//active[fromLeft + 1]
active[fromLeft + 2] = (active[fromLeft + 1] >> 1);
updateColumn(fromLeft + 2);
fromLeft++;
krok++;
angle = 3;
}
break;
case 3:
// . o . . . .
// . o o ---> . o o
// . . o o o .
if((fromLeft > 0)
&& ((active[fromLeft + 1] & ((active[fromLeft + 1] >> 1)) & screen[fromLeft - 1]) == 0)
&& ((active[fromLeft + 1] & screen[fromLeft]) == 0))
{
active[fromLeft - 1] = (active[fromLeft + 1] & (active[fromLeft + 1] >> 1));
updateColumn(fromLeft - 1);
active[fromLeft] = active[fromLeft + 1];
updateColumn(fromLeft);
active[fromLeft + 1] = (active[fromLeft - 1] << 1);
updateColumn(fromLeft + 1);
fromLeft--;
angle = 0;
}
break;
}
break;
}
playNote('E', 10);
buttonDelay(200);
}
}
//Restart
if(digitalRead(start_button) == LOW)
{
memset(lc0, 0, sizeof(lc0));
memset(lc1, 0, sizeof(lc1));
memset(active, 0, sizeof(active));
memset(screen, 0, sizeof(screen));
score = 0;
allLines = 0;
figura = 0;
break;
}
for(int colnum = 0; colnum < lcCols; colnum++)
{
if((screen[colnum] & (active[colnum] >> 1)) == 0)
{
colCheck++;
}
else
{
colCheck = 0;
if(krok == 0)
{
started = 0;
}
}
}
if((colCheck == lcCols) && (krok < lcRows))
{
for(int colnum = 0; colnum < lcCols; colnum++)
{
active[colnum] = active[colnum] >> 1;
updateColumn(colnum);
}
}
else
{
break;
}
delay(brickDelay);
}
for(int colnum = 0; colnum < lcCols; colnum++)
{
screen[colnum] = screen[colnum] | (lc0[colnum] << (lcRows / 2));
screen[colnum] = screen[colnum] | lc1[colnum];
lc0[colnum] = 0;
lc1[colnum] = 0;
active[colnum] = 0;
}
currLines = 0;
for(int rownum = 0; rownum < lcRows; rownum++)
{
colCheck = 0;
for(int colnum = 0; colnum < lcCols; colnum++)
{
if(((screen[colnum] >> rownum) & 1) == 1)
{
colCheck++;
}
}
if(colCheck == lcCols)
{
//Animacja kasowania
for(int colnum = 0; colnum < lcCols; colnum++)
{
tmpCol = ~((int) round(pow(2, rownum)));
screen[colnum] = screen[colnum] & tmpCol;
updateColumn(colnum);
switch(currLines)
{
case 0:
playNote('b', 20);
break;
case 1:
playNote('D', 20);
break;
case 2:
playNote('F', 20);
break;
case 3:
playNote('A', 20);
break;
}
delay(30);
tmpCol = (int) (round(pow(2, rownum)) - 1);
tmpCol = screen[colnum] & tmpCol;
screen[colnum] = (screen[colnum] >> (rownum + 1));
screen[colnum] = (screen[colnum] << rownum);
screen[colnum] = screen[colnum] | tmpCol;
}
for(int colnum = 0; colnum < lcCols; colnum++)
{
updateColumn(colnum);
}
rownum--;
currLines++;
allLines++;
}
}
if(currLines > 0)
{
score += (int) round(pow(4, currLines-1));
}
}
gameOver();
// == Game Over ==
}
}
void gameOver()
{
playNote('F', 80);
playNote('A', 60);
playNote('F', 80);
playNote('A', 60);
int cima[] =
{
B11111111, // o o o o
B11111111, // o o o o
B11111111, // o o o o
B11111111, // o o
B11111111, // o o o o
B11111111, //
B11111111, // o o o o o o
B11111111 // o o o
};
int baixo[] =
{
B11111111, // o o o
B11111111, // o o o
B11111111, // o o o
B11111111, //
B11111111, // o o o o
B11111111, // o o
B11111111, // o o
B11111111 // o o o o
};
for(int rownum = 8; rownum >= 0; rownum--)
{
lc.setRow(1,rownum,baixo[rownum]);
delay(100);
}
for(int rownum = 8; rownum >= 0; rownum--)
{
lc.setRow(0,rownum,cima[rownum]);
delay(100);
}
delay(1800);
}
6. Testing and Debugging
6.1 Checking Display Output
- Ensure the LED matrix lights up correctly.
- Use a basic LED test program to confirm wiring is correct.
6.2 Verifying Controls
- Read joystick values using Serial Monitor.
- Confirm rotation and movement logic works.
6.3 Troubleshooting Issues
- Random screen glitches? Check power supply stability.
- Joystick not responding? Verify analog pin readings.
- Blocks not rotating? Ensure button is wired correctly.
7. Advanced Features
7.1 Adding Sound Effects
- Connect a buzzer to an Arduino pin.
- Play tones for movement, rotation, and line clearing.
7.2 Implementing High Scores
- Use EEPROM to store and retrieve scores.
- Display high score at the start of the game.
7.3 Multi-Matrix Expansion
- Connect multiple Max7219 displays for a bigger game area.
- Update code to handle a larger grid.
8. Real-World Applications
- STEM education projects – Teaching game logic & hardware programming.
- Retro gaming projects – Reviving classic games on DIY hardware.
- Prototyping interactive LED displays – Beyond gaming, LED matrices can be used for animations and digital signage.
9. FAQs
9.1 Can I use an Arduino Nano instead of an Uno?
Yes, but ensure it has enough I/O pins and supports SPI communication.
9.2 How can I make the game run faster?
Optimize game logic and reduce display refresh delays.
9.3 Can I add a second player?
Yes, by using two joysticks and modifying game logic for multiplayer mode.
9.4 Why is my display not working?
Check Max7219 connections, ensure CS, DIN, and CLK are correctly wired.
9.5 How do I expand the game grid?
Chain multiple Max7219 modules and modify LED mapping in code.
10. Conclusion
The Arduino Tetris Game with Max7219 8×8 LED Matrix Display is an engaging project that merges hardware programming with game development. It enhances coding skills, understanding of SPI communication, and real-time game logic implementation. Experiment with additional features like sound, high scores, and multiplayer mode to make it even more fun!
11. Arduino Projects:
1- Complete Guide for DHT11/DHT22 Humidity and Temperature Sensor With Arduino
2- DHT11 – Temperature and Humidity Sensor
3- DHT22 – Temperature and Humidity Sensor (more accurate than DHT11)
4- BMP180 – Barometric Pressure and Altitude Sensor
5- BMP280 – Barometric Pressure & Temperature Sensor
6- BME280 – Temperature, Humidity, and Pressure Sensor
7- Arduino Flex Sensor Controlled Robot Hand
8- Arduino ECG Heart Rate Monitor AD8232 Demo
9- Arduino NRF24L01 Wireless Joystick Robot Car
10- Arduino Force Sensor Anti-Theft Alarm System
11- Arduino NRF24L01 Transceiver Controlled Relay Light
12- Arduino Rotary Encoder Controlled LEDs: A Complete Guide