Table of Contents
1. Introduction
The world is rapidly moving towards touchless technology, and gesture-controlled interfaces are becoming increasingly popular. From smartphones to home automation, touch-free systems provide convenience, accessibility, and hygiene benefits. One exciting application of this technology is an Arduino-based elevator system that uses the APDS-9960 sensor for gesture recognition.
1.1 Overview of Gesture-Controlled Systems
Gesture-controlled systems rely on sensors to detect hand movements and interpret them as commands. These systems eliminate the need for physical touch, making them ideal for environments where hygiene is crucial, such as hospitals, offices, and public spaces.
1.2 Importance of Contactless Interfaces in Modern Elevators
In recent years, there has been a surge in the demand for contactless technology due to concerns about hygiene and efficiency. Traditional elevator buttons can harbor germs, making gesture-based controls a safer alternative.
To understand more about the impact of contactless technology, check out this guide on smart interfaces.
1.3 Introduction to the APDS-9960 Sensor
The APDS-9960 sensor is a compact, multifunctional device that provides gesture recognition, proximity sensing, ambient light detection, and color sensing. It operates using infrared light to track hand movements, making it perfect for gesture-controlled elevator systems.
For a deeper dive into the technical aspects of this sensor, visit this technical breakdown of the APDS-9960.
2. Understanding the APDS-9960 Sensor
2.1 Features and Specifications
The APDS-9960 sensor offers several advanced features that make it ideal for gesture-controlled applications:
- Gesture Recognition – Detects directional hand movements (left, right, up, down).
- Proximity Detection – Measures the distance between an object and the sensor.
- Ambient Light Sensing – Adjusts brightness based on surrounding light conditions.
- Color Sensing – Identifies colors using red, green, and blue (RGB) detection.
2.2 Working Principle
The sensor emits infrared (IR) light and measures the reflected signals to detect movement. The Arduino processes these signals and translates them into commands. For example, a swipe-up motion can indicate an elevator call, while a swipe-right can select a floor.
2.3 Applications Beyond Elevators
Apart from elevators, gesture recognition with the APDS-9960 is used in:
- Smart Home Automation – Lights, TVs, and appliances controlled via gestures.
- Gaming Systems – Hands-free controls for an immersive experience.
- Industrial Control Panels – Reducing physical contact in hazardous environments.
3. Components Required for the Gesture-Controlled Elevator System
3.1 Arduino Board Selection
The Arduino Uno is the preferred choice due to its ease of use and widespread support. However, other compatible options include:
- Arduino Nano – Suitable for compact installations.
- Arduino Mega – Ideal for projects requiring multiple sensor integrations.
3.2 APDS-9960 Gesture Sensor
The APDS-9960 sensor module must be properly mounted and positioned for accurate gesture recognition.
3.3 Display Modules
For user feedback, an OLED or LCD display can show the elevator’s current status and received commands.
3.4 Motor and Motor Driver
A stepper motor or DC motor with a motor driver (L298N or A4988) is required to operate the elevator mechanism.
3.5 Additional Components
- Power supply (5V or 12V based on motor requirements).
- Jumper wires for circuit connections.
- Enclosure for housing the system securely.
4. System Design and Architecture
4.1 Functional Block Diagram
The system consists of the following key connections:
- APDS-9960 sensor → Detects gestures.
- Arduino → Processes input and sends signals.
- Motor driver → Controls the motor based on received commands.
- LCD display → Provides user feedback.
4.2 Gesture Mapping to Elevator Controls
- Swipe Up → Move elevator up.
- Swipe Down → Move elevator down.
- Swipe Right → Open doors.
- Swipe Left → Close doors.
4.3 Safety Considerations
- Implementing a timeout mechanism to prevent accidental activation.
- Proximity sensors to detect if a person is standing in the doorway.
5. Circuit Design and Wiring
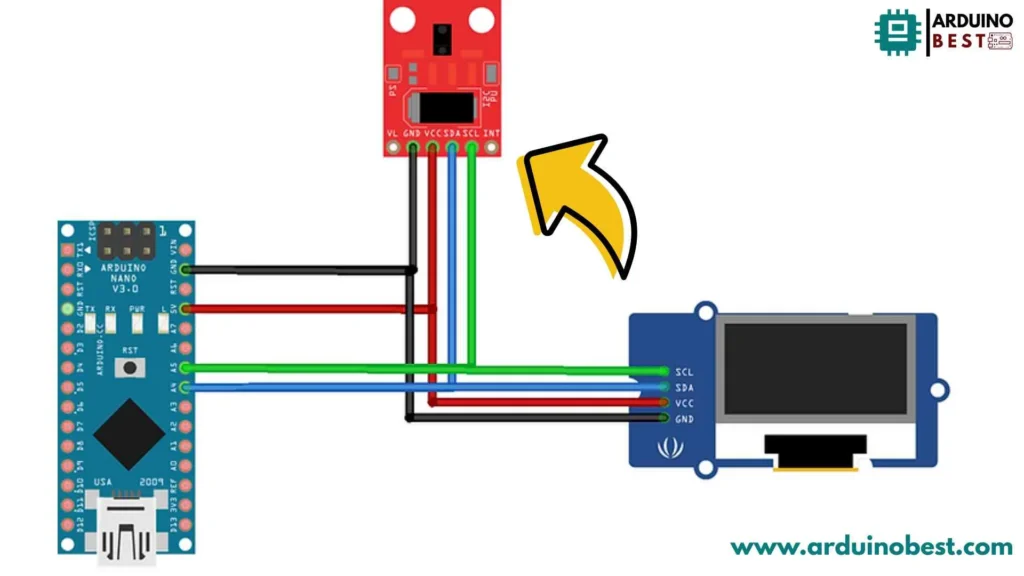
5.1 Connecting the APDS-9960 Sensor to Arduino
- VCC → 3.3V (Do not use 5V to avoid damaging the sensor).
- GND → Ground.
- SDA & SCL → I2C communication pins on Arduino.
- INT → Interrupt pin for gesture detection.
5.2 Integrating the Display Module
- LCD/OLED Display → Connected via I2C for real-time updates.
5.3 Motor and Driver Circuitry
- Motor driver connected to Arduino’s digital output pins.
- Stepper motor or DC motor receives signals from the motor driver to move the elevator.
6. Programming the Arduino
6.1 Installing Necessary Libraries
cppCopyEdit#include <Wire.h>
#include <Adafruit_APDS9960.h>
#include <LiquidCrystal_I2C.h>
6.2 Writing the Gesture Recognition Code
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SH1106.h>
#include <Arduino_APDS9960.h>
#define OLED_RESET -1
Adafruit_SH1106 display(OLED_RESET);
int floornum=0;
int currentfloor=0;
//Paste your bitmap here
const unsigned char up [] PROGMEM = {
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x38, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7c, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0xff, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x03, 0xff, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x0f, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x1f, 0xff, 0xf0,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xff, 0xfc, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xff, 0xff, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x03, 0xff, 0xc7, 0xff, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x07, 0xff, 0x81, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x1f, 0xfe, 0x00,
0xff, 0xf0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x3f, 0xfc, 0x00, 0x3f, 0xf8, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xff, 0xf0, 0x00, 0x1f, 0xfe, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x01, 0xff, 0xe0, 0x00, 0x07, 0xff, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x07, 0xff, 0x80, 0x00, 0x03, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0f, 0xff, 0x00,
0x00, 0x00, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x3f, 0xfc, 0x00, 0x00, 0x00, 0x7f,
0xf8, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xf8, 0x00, 0x00, 0x00, 0x1f, 0xfc, 0x00, 0x00,
0x00, 0x00, 0x00, 0x01, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x0f, 0xff, 0x00, 0x00, 0x00, 0x00, 0x00,
0x03, 0xff, 0xc0, 0x00, 0x38, 0x00, 0x03, 0xff, 0x80, 0x00, 0x00, 0x00, 0x00, 0x0f, 0xff, 0x00,
0x00, 0x7c, 0x00, 0x01, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x1f, 0xfe, 0x00, 0x01, 0xff, 0x00,
0x00, 0x7f, 0xf0, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xf8, 0x00, 0x03, 0xff, 0x80, 0x00, 0x3f, 0xfc,
0x00, 0x00, 0x00, 0x00, 0xff, 0xf0, 0x00, 0x0f, 0xff, 0xe0, 0x00, 0x0f, 0xfe, 0x00, 0x00, 0x00,
0x03, 0xff, 0xc0, 0x00, 0x1f, 0xff, 0xf0, 0x00, 0x07, 0xff, 0x80, 0x00, 0x00, 0x07, 0xff, 0x80,
0x00, 0x7f, 0xff, 0xfc, 0x00, 0x01, 0xff, 0xc0, 0x00, 0x00, 0x1f, 0xfe, 0x00, 0x00, 0xff, 0xff,
0xfe, 0x00, 0x00, 0xff, 0xf0, 0x00, 0x00, 0x3f, 0xfc, 0x00, 0x03, 0xff, 0xc7, 0xff, 0x80, 0x00,
0x3f, 0xf8, 0x00, 0x00, 0xff, 0xf0, 0x00, 0x07, 0xff, 0x81, 0xff, 0xc0, 0x00, 0x1f, 0xfe, 0x00,
0x01, 0xff, 0xe0, 0x00, 0x1f, 0xfe, 0x00, 0xff, 0xf0, 0x00, 0x07, 0xff, 0x00, 0x07, 0xff, 0x80,
0x00, 0x3f, 0xfc, 0x00, 0x3f, 0xf8, 0x00, 0x03, 0xff, 0xc0, 0x0f, 0xfe, 0x00, 0x00, 0xff, 0xf0,
0x00, 0x1f, 0xfe, 0x00, 0x00, 0xff, 0xe0, 0x3f, 0xfc, 0x00, 0x01, 0xff, 0xe0, 0x00, 0x07, 0xff,
0x00, 0x00, 0x7f, 0xf0, 0x1f, 0xf0, 0x00, 0x07, 0xff, 0x80, 0x00, 0x03, 0xff, 0xc0, 0x00, 0x1f,
0xf0, 0x0f, 0xe0, 0x00, 0x0f, 0xff, 0x00, 0x00, 0x00, 0xff, 0xe0, 0x00, 0x0f, 0xe0, 0x03, 0xc0,
0x00, 0x3f, 0xfc, 0x00, 0x00, 0x00, 0x7f, 0xf8, 0x00, 0x03, 0x80, 0x01, 0x00, 0x00, 0x7f, 0xf8,
0x00, 0x00, 0x00, 0x1f, 0xfc, 0x00, 0x01, 0x00, 0x00, 0x00, 0x01, 0xff, 0xe0, 0x00, 0x00, 0x00,
0x0f, 0xff, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x03, 0xff, 0x80,
0x00, 0x00, 0x00, 0x00, 0x0f, 0xff, 0x00, 0x00, 0x00, 0x00, 0x01, 0xff, 0xe0, 0x00, 0x00, 0x00,
0x00, 0x1f, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xf0, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xf8,
0x00, 0x00, 0x00, 0x00, 0x00, 0x3f, 0xfc, 0x00, 0x00, 0x00, 0x00, 0xff, 0xf0, 0x00, 0x00, 0x00,
0x00, 0x00, 0x0f, 0xfe, 0x00, 0x00, 0x00, 0x03, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x07,
0xff, 0x80, 0x00, 0x00, 0x07, 0xff, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0xff, 0xc0, 0x00,
0x00, 0x1f, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xff, 0xf0, 0x00, 0x00, 0x3f, 0xfc,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x3f, 0xf8, 0x00, 0x00, 0xff, 0xf0, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x1f, 0xfe, 0x00, 0x01, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x07, 0xff, 0x00, 0x07, 0xff, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xff,
0xc0, 0x0f, 0xff, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xff, 0xe0, 0x3f, 0xfc,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xf0, 0x1f, 0xf0, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x1f, 0xf0, 0x0f, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x0f, 0xe0, 0x03, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x03, 0x80, 0x01, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00
};
const unsigned char down [] PROGMEM = {
// 'down, 105x64px
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x40, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x40, 0x00, 0xe0,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0xe0, 0x03, 0xf8, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xf0, 0x0f, 0xfc, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x07, 0xf0, 0x0f, 0xff, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x1f, 0xf0, 0x03, 0xff, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xf0, 0x01,
0xff, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xff, 0xf0, 0x00, 0x7f, 0xf0, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0xff, 0xc0, 0x00, 0x3f, 0xfc, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x07, 0xff, 0x80, 0x00, 0x0f, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x1f, 0xfe, 0x00, 0x00, 0x07, 0xff, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x3f, 0xfc, 0x00,
0x00, 0x01, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xff, 0xf0, 0x00, 0x00, 0x00, 0xff,
0xf0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x3f, 0xf8, 0x00, 0x00,
0x00, 0x00, 0x00, 0x07, 0xff, 0x80, 0x00, 0x00, 0x00, 0x1f, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x00,
0x0f, 0xff, 0x00, 0x00, 0x00, 0x00, 0x07, 0xff, 0x00, 0x00, 0x00, 0x00, 0x00, 0x3f, 0xfc, 0x00,
0x00, 0x00, 0x00, 0x03, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xf8, 0x00, 0x00, 0x00, 0x00,
0x00, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x01, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xf8,
0x00, 0x00, 0x00, 0x03, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x40, 0x00, 0x1f, 0xfc, 0x00, 0x00, 0x00,
0x0f, 0xff, 0x00, 0x00, 0x40, 0x00, 0xe0, 0x00, 0x0f, 0xff, 0x00, 0x00, 0x00, 0x1f, 0xfe, 0x00,
0x01, 0xe0, 0x03, 0xf8, 0x00, 0x03, 0xff, 0x80, 0x00, 0x00, 0x7f, 0xf8, 0x00, 0x03, 0xf0, 0x0f,
0xfc, 0x00, 0x01, 0xff, 0xe0, 0x00, 0x00, 0xff, 0xf0, 0x00, 0x07, 0xf0, 0x0f, 0xff, 0x00, 0x00,
0x7f, 0xf0, 0x00, 0x03, 0xff, 0xc0, 0x00, 0x1f, 0xf0, 0x03, 0xff, 0x80, 0x00, 0x3f, 0xfc, 0x00,
0x07, 0xff, 0x80, 0x00, 0x3f, 0xf0, 0x01, 0xff, 0xe0, 0x00, 0x0f, 0xfe, 0x00, 0x1f, 0xfe, 0x00,
0x00, 0xff, 0xf0, 0x00, 0x7f, 0xf0, 0x00, 0x07, 0xff, 0x80, 0x3f, 0xfc, 0x00, 0x03, 0xff, 0xc0,
0x00, 0x3f, 0xfc, 0x00, 0x01, 0xff, 0xc0, 0xff, 0xf0, 0x00, 0x07, 0xff, 0x80, 0x00, 0x0f, 0xfe,
0x00, 0x00, 0xff, 0xf1, 0xff, 0xe0, 0x00, 0x1f, 0xfe, 0x00, 0x00, 0x07, 0xff, 0x80, 0x00, 0x3f,
0xff, 0xff, 0x80, 0x00, 0x3f, 0xfc, 0x00, 0x00, 0x01, 0xff, 0xc0, 0x00, 0x1f, 0xff, 0xff, 0x00,
0x00, 0xff, 0xf0, 0x00, 0x00, 0x00, 0xff, 0xf0, 0x00, 0x07, 0xff, 0xfc, 0x00, 0x01, 0xff, 0xe0,
0x00, 0x00, 0x00, 0x3f, 0xf8, 0x00, 0x03, 0xff, 0xf8, 0x00, 0x07, 0xff, 0x80, 0x00, 0x00, 0x00,
0x1f, 0xfe, 0x00, 0x00, 0xff, 0xe0, 0x00, 0x0f, 0xff, 0x00, 0x00, 0x00, 0x00, 0x07, 0xff, 0x00,
0x00, 0x7f, 0xc0, 0x00, 0x3f, 0xfc, 0x00, 0x00, 0x00, 0x00, 0x03, 0xff, 0xc0, 0x00, 0x1f, 0x00,
0x00, 0x7f, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x00, 0xff, 0xe0, 0x00, 0x0e, 0x00, 0x01, 0xff, 0xe0,
0x00, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xf8, 0x00, 0x00, 0x00, 0x03, 0xff, 0xc0, 0x00, 0x00, 0x00,
0x00, 0x00, 0x1f, 0xfc, 0x00, 0x00, 0x00, 0x0f, 0xff, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0f,
0xff, 0x00, 0x00, 0x00, 0x1f, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xff, 0x80, 0x00,
0x00, 0x7f, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0xff, 0xe0, 0x00, 0x00, 0xff, 0xf0,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xf0, 0x00, 0x03, 0xff, 0xc0, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x3f, 0xfc, 0x00, 0x07, 0xff, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x0f, 0xfe, 0x00, 0x1f, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x07, 0xff,
0x80, 0x3f, 0xfc, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0xff, 0xc0, 0xff, 0xf0,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xff, 0xf1, 0xff, 0xe0, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x3f, 0xff, 0xff, 0x80, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x1f, 0xff, 0xff, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x07, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xff, 0xf8,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xff, 0xe0, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x1f, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x0e, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00
};
const unsigned char uparrow [] PROGMEM = {
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x0e, 0x00, 0x00, 0x00, 0x00, 0x1f, 0x00, 0x00, 0x00, 0x00, 0x3f, 0x80, 0x00, 0x00, 0x00,
0xff, 0xc0, 0x00, 0x00, 0x01, 0xff, 0xf0, 0x00, 0x00, 0x03, 0xff, 0xf8, 0x00, 0x00, 0x07, 0xff,
0xfc, 0x00, 0x00, 0x1f, 0xff, 0xff, 0x00, 0x00, 0x3f, 0xff, 0xff, 0x80, 0x00, 0xff, 0xff, 0xff,
0xc0, 0x01, 0xff, 0xff, 0xff, 0xf0, 0x03, 0xff, 0xff, 0xff, 0xf8, 0x03, 0xff, 0xff, 0xff, 0xf8,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00
};
const unsigned char downarrow [] PROGMEM = {
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x1f, 0xff, 0xff, 0xff, 0xc0, 0x1f,
0xff, 0xff, 0xff, 0xc0, 0x0f, 0xff, 0xff, 0xff, 0x80, 0x03, 0xff, 0xff, 0xff, 0x00, 0x01, 0xff,
0xff, 0xfc, 0x00, 0x00, 0xff, 0xff, 0xf8, 0x00, 0x00, 0x3f, 0xff, 0xe0, 0x00, 0x00, 0x1f, 0xff,
0xc0, 0x00, 0x00, 0x0f, 0xff, 0x80, 0x00, 0x00, 0x03, 0xff, 0x00, 0x00, 0x00, 0x01, 0xfc, 0x00,
0x00, 0x00, 0x00, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x70, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00
};
const unsigned char dooropen [] PROGMEM = {
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0f, 0xff, 0xff, 0xc0, 0x1f, 0xff, 0xff, 0xe0,
0x3f, 0xff, 0xff, 0xe0, 0x3f, 0xff, 0xff, 0xf0, 0x38, 0x00, 0x00, 0xf0, 0x38, 0x00, 0x00, 0xf0,
0x38, 0x00, 0x00, 0xf0, 0x38, 0x04, 0x80, 0xf0, 0x38, 0x1c, 0xc0, 0xf0, 0x38, 0x3c, 0xf0, 0xf0,
0x38, 0x7c, 0xf8, 0xf0, 0x39, 0xfc, 0xfc, 0xf0, 0x3b, 0xfc, 0xfe, 0xf0, 0x3b, 0xfc, 0xfe, 0xf0,
0x38, 0xfc, 0xfc, 0xf0, 0x38, 0x7c, 0xf8, 0xf0, 0x38, 0x3c, 0xf0, 0xf0, 0x38, 0x1c, 0xc0, 0xf0,
0x38, 0x04, 0x80, 0xf0, 0x38, 0x00, 0x00, 0xf0, 0x38, 0x00, 0x00, 0xf0, 0x38, 0x00, 0x00, 0xf0,
0x3c, 0x00, 0x00, 0xf0, 0x3f, 0xff, 0xff, 0xe0, 0x1f, 0xff, 0xff, 0xe0, 0x1f, 0xff, 0xff, 0xc0,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00
};
const unsigned char closedoor [] PROGMEM = {
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x0f, 0xff, 0xff, 0xc0, 0x0f, 0xff, 0xff, 0xc0, 0x08, 0x00, 0x00, 0x40, 0x0a, 0x00, 0x03, 0x40,
0x0b, 0x80, 0x07, 0x40, 0x0b, 0xc0, 0x0f, 0x40, 0x0b, 0xe0, 0x1f, 0x40, 0x0b, 0xf0, 0x3f, 0x40,
0x0b, 0xf8, 0x7f, 0x40, 0x0b, 0xfc, 0xff, 0x40, 0x0b, 0xff, 0xff, 0x40, 0x0b, 0xff, 0xff, 0x40,
0x0b, 0xfe, 0xff, 0x40, 0x0b, 0xf8, 0x7f, 0x40, 0x0b, 0xf0, 0x3f, 0x40, 0x0b, 0xe0, 0x1f, 0x40,
0x0b, 0xc0, 0x0f, 0x40, 0x0b, 0x80, 0x07, 0x40, 0x0b, 0x00, 0x03, 0x40, 0x08, 0x00, 0x01, 0x40,
0x0f, 0xff, 0xff, 0xc0, 0x0f, 0xff, 0xff, 0xc0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00
};
void setup(){
Serial.begin(9600);
if (!APDS.begin()) {
Serial.println("Error initializing APDS9960 sensor!");
}
Serial.println("Detecting gestures ...");
display.begin(SH1106_SWITCHCAPVCC, 0x3C);
display.setTextSize(2);
display.setTextColor(WHITE);
display.clearDisplay();
display.display();
home1();
}
void loop() {
display.clearDisplay();
if (APDS.gestureAvailable()) {
int gesture = APDS.readGesture();
switch (gesture) {
case GESTURE_UP:
Serial.println("Detected UP gesture");
display.clearDisplay();
floornum ++;
home1();
break;
case GESTURE_DOWN:
Serial.println("Detected DOWN gesture");
display.clearDisplay();
floornum --;
home1();
break;
case GESTURE_LEFT:
Serial.println("Detected LEFT gesture");
display.clearDisplay();
start();
break;
case GESTURE_RIGHT:
Serial.println("Detected RIGHT gesture");
display.clearDisplay();
home1();
break;
default:
break;
}
}
}
void home1()
{
display.setCursor(101,23);
display.println(floornum);
display.drawBitmap(23, 0, uparrow, 40, 18, WHITE);
display.drawBitmap(26, 46, downarrow, 40, 18, WHITE);
display.drawBitmap(0, 15, dooropen, 29, 30, WHITE);
display.drawBitmap(60, 15, closedoor, 29, 30, WHITE);
display.display();
}
void start()
{
while(floornum > currentfloor){
Serial.println("going UP ");
currentfloor++;
display.drawBitmap(0, 0, up, 100, 64, WHITE);
display.setCursor(101,23);
display.println(currentfloor);
display.display();
display.clearDisplay();
delay(2000);
}
while(floornum < currentfloor){
Serial.println("going Down ");
currentfloor--;
display.drawBitmap(0, 0, down, 100, 64, WHITE);
display.setCursor(101,23);
display.println(currentfloor);
display.display();
display.clearDisplay();
delay(2000);
}
if(floornum== currentfloor){
Serial.println("Reached");
display.clearDisplay();
home1();
Serial.print(currentfloor);
}
}
6.3 Implementing Elevator Control Logic
Modify the loop to integrate motor movement based on detected gestures.
7. Testing and Calibration
7.1 Initial System Testing
- Power up and check if the gesture sensor is responding correctly.
- Verify the motor movement in response to gestures.
7.2 Gesture Recognition Calibration
- Adjust sensor placement to optimize detection.
- Test under different lighting conditions to ensure reliability.
7.3 Troubleshooting Common Issues
- Sensor not responding? Check I2C connections.
- Elevator not moving? Verify motor driver wiring and power supply.
8. Advanced Features and Enhancements
8.1 Adding Voice Control Integration
- Integrate voice recognition modules like Elechouse V3 for voice + gesture control.
8.2 Remote Monitoring and Control
- Use Wi-Fi or Bluetooth modules (ESP8266, HC-05) for smartphone control.
8.3 User Authentication Mechanisms
- Implement RFID or Face Recognition for access control.
9. Applications and Use Cases
9.1 Residential Buildings
- Provides a hygienic and futuristic solution for apartment complexes.
9.2 Commercial Establishments
- Reduces wear-and-tear on physical buttons in high-traffic areas.
9.3 Accessibility Improvements
- Enhances elevator accessibility for individuals with physical disabilities.
10. FAQs
10.1 How does the APDS-9960 sensor detect gestures?
It uses infrared light reflections to track hand movements.
10.2 Can this system be integrated into existing elevators?
Yes, it can be adapted by interfacing with the elevator’s control system.
10.3 What is the range of the APDS-9960 sensor?
Between 5cm and 30cm, depending on ambient light.
11. Conclusion
The Arduino Gesture APDS-9960 Sensor Elevator System offers a smart, touchless solution that enhances safety, convenience, and hygiene. With future improvements like voice control and IoT integration, gesture-controlled elevators can revolutionize the way we interact with buildings.
12. Arduino Projects:
1- Complete Guide for DHT11/DHT22 Humidity and Temperature Sensor With Arduino
2- DHT11 – Temperature and Humidity Sensor
3- DHT22 – Temperature and Humidity Sensor (more accurate than DHT11)
4- BMP180 – Barometric Pressure and Altitude Sensor
5- BMP280 – Barometric Pressure & Temperature Sensor
6- BME280 – Temperature, Humidity, and Pressure Sensor
7- Arduino Flex Sensor Controlled Robot Hand
8- Arduino ECG Heart Rate Monitor AD8232 Demo
9- Arduino NRF24L01 Wireless Joystick Robot Car
10- Arduino Force Sensor Anti-Theft Alarm System
11- Arduino NRF24L01 Transceiver Controlled Relay Light
12- Arduino Rotary Encoder Controlled LEDs: A Complete Guide